Sample Code:
HTML:
<template>
<lightning-card title="Prechat Form">
<template class="slds-m-around_medium" for:each={fields} for:item="field">
<template if:true={field.dropDown} key={field.name}>
<div key={field.name}>Priority</div>
<select class="slds-select" key={field.name} onchange={handlePriorityChange}>
<option label="Low" value="Low"></option>
<option label="Medium" value="Medium"></option>
<option label="High" value="High"></option>
</select>
</template>
<template if:false={field.dropDown} key={field.name}>
<lightning-input
key={field.name}
name={field.name}
label={field.label}
value={field.value}
max-length={field.maxLength}
required={field.required}>
</lightning-input>
</template>
</template>
</lightning-card>
<br/>
<lightning-button
label="Start Chat"
title="Start Chat"
onclick={handleStartChat}
class="slds-m-left_x-small"
variant="brand">
</lightning-button>
</template>
JavaScript:
import BasePrechat from 'lightningsnapin/basePrechat';
import { api } from 'lwc';
export default class Prechat extends BasePrechat {
@api prechatFields;
fields;
namelist;
priorityValue = 'Low';
get priorityOptions() {
return [
{ label: 'Low', value: 'Low' },
{ label: 'Medium', value: 'Medium' },
{ label: 'High', value: 'High' },
];
}
handlePriorityChange( event ) {
console.log( 'Updated Value is', JSON.stringify( event.target.value ) );
this.priorityValue = event.target.value;
}
/**
* Set the button label and prepare the prechat fields to be shown in the form.
*/
connectedCallback() {
this.fields = this.prechatFields.map( field => {
console.log( 'Field is ', JSON.stringify( field ) );
let dropDown = false;
if ( field.label == 'Priority' ) {
dropDown = true;
}
const { label, name, value, required, maxLength } = field;
return { label, value, name, required, maxLength, dropDown };
});
this.namelist = this.fields.map( field => field.name );
}
/**
* On clicking the 'Start Chatting' button, send a chat request.
*/
handleStartChat() {
this.template.querySelectorAll( "lightning-input" ).forEach( input => {
this.fields[ this.namelist.indexOf( input.name ) ].value = input.value;
} );
this.fields[ this.namelist.indexOf( 'Priority' ) ].value = this.priorityValue;
console.log( 'Updated Fields values are', JSON.stringify( this.fields ) );
this.startChat( this.fields );
}
}
JS-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>52.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightningSnapin__PreChat</target>
</targets>
</LightningComponentBundle>
Output:
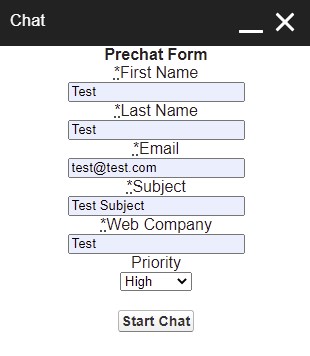