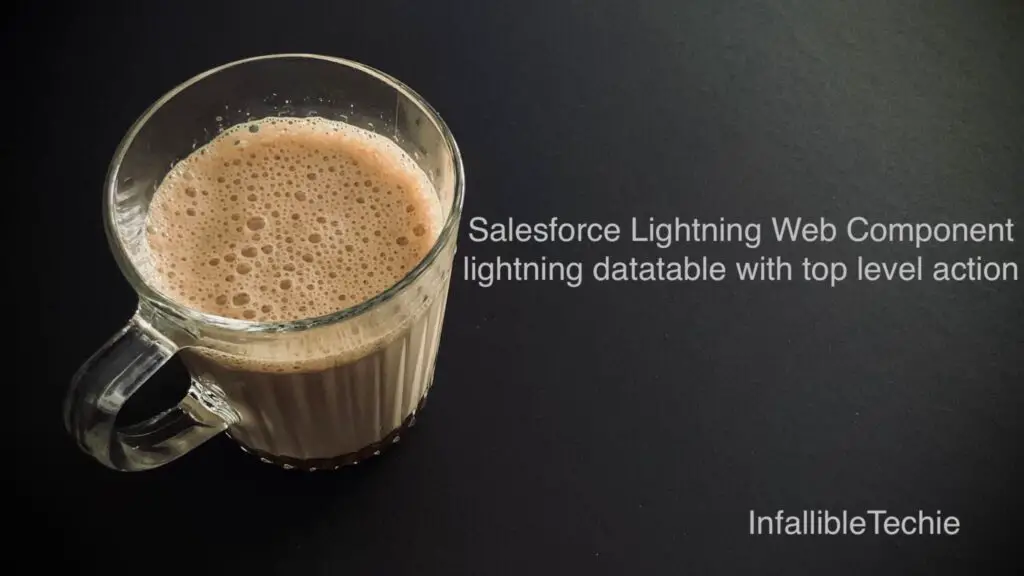
We can use lightnging-card and lightning/navigation to show New button as top level action along with lightning-datatable.
Sample Code:
Apex Class:
public class AccountController {
@AuraEnabled( cacheable = true )
public static List< Account > fetchAccounts() {
return [
SELECT Id, Name, Industry
FROM Account
LIMIT 10
];
}
}
Lightning Web Component:
HTML:
<template>
<lightning-card title="Accounts" icon-name="standard:account">
<div slot="actions">
<lightning-button-menu
alternative-text="Show menu"
onselect={handleOnselect}>
<lightning-menu-item
value="New"
label="New">
</lightning-menu-item>
</lightning-button-menu>
</div>
<template if:true={accounts}>
<lightning-datatable
key-field="Id"
data={accounts}
columns={columns}
onrowaction={handleRowAction}
onrowselection={handleRowSelection}>
</lightning-datatable>
</template>
<template if:true={error}>
{error}>
</template>
</lightning-card>
</template>
import { LightningElement, wire } from 'lwc';
import fetchAccounts from '@salesforce/apex/AccountController.fetchAccounts';
import { NavigationMixin } from 'lightning/navigation';
export default class sampleLightningWebComponent extends NavigationMixin( LightningElement ) {
accounts;
error;
selectedAccounts = [];
columns = [
{ label: 'Name', fieldName: 'Name' },
{ label: 'Industry', fieldName: 'Industry' },
{
type: 'action',
typeAttributes: { rowActions: this.getRowActions.bind( this ) }
}
];
@wire( fetchAccounts )
wiredAccount( { error, data } ) {
if ( data ) {
this.accounts = data;
this.error = undefined;
} else if ( error ) {
this.error = error;
this.accounts = undefined;
}
}
getRowActions( row, doneCallback ) {
const actions = [];
if ( this.selectedAccounts && this.selectedAccounts.includes( row[ 'Id' ] ) ) {
actions.push( {
'label': 'View',
'name': 'view'
} );
actions.push( {
'label': 'Edit',
'name': 'edit'
} );
}
setTimeout( () => {
doneCallback( actions );
}, 200 );
}
handleRowAction( event ) {
const actionName = event.detail.action.name;
const row = event.detail.row;
switch ( actionName ) {
case 'view':
this[ NavigationMixin.Navigate ] ( {
type: 'standard__recordPage',
attributes: {
recordId: row.Id,
actionName: 'view'
}
} );
break;
case 'edit':
this[ NavigationMixin.Navigate ] ( {
type: 'standard__recordPage',
attributes: {
recordId: row.Id,
objectApiName: 'Account',
actionName: 'edit'
}
});
break;
default:
}
}
handleRowSelection( event ) {
const selectedRows = event.detail.selectedRows;
for ( let i = 0; i < selectedRows.length; i++ ) {
this.selectedAccounts.push( selectedRows[ i ].Id );
}
}
handleOnselect( event ) {
let selectedAction = event.detail.value;
if ( selectedAction == 'New' ) {
console.log( 'Inside Account Creation' );
this[ NavigationMixin.Navigate ] ( {
type: 'standard__objectPage',
attributes: {
objectApiName: 'Account',
actionName: 'new'
}
} );
}
}
}
js-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>57.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__Tab</target>
</targets>
</LightningComponentBundle>
Output:
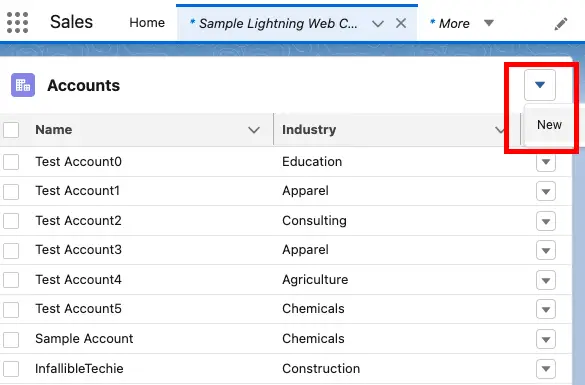