Gain granular control over the look and feel of your Salesforce Messaging for Web chat interactions. The lightningSnapin__MessagingTextMessageBubble target facilitates the inclusion of Lightning Web Components, providing a mechanism to style and transform how messages are displayed.
Sample Lightning Web Component:
HTML:
<template>
<div class={generateMessageBubbleClassname}>
<lightning-formatted-rich-text
value={textContent}>
</lightning-formatted-rich-text>
</div>
</template>
JavaScript:
import { api, LightningElement } from "lwc";
/* Contstants */
const AGENT = "Agent";
const ENDUSER = "EndUser";
const CHATBOT = "Chatbot";
const PARTICIPANT_TYPES = [ ENDUSER, AGENT, CHATBOT ];
const MESSAGE_CONTENT_CLASS = "embedded-messaging-message-content";
export default class MessagingChatBubble extends LightningElement {
/**
* Deployment configuration data.
* @type {Object}
*/
@api configuration;
/**
* Conversation entry data.
* @type {Object}
*/
@api conversationEntry;
/**
* Returns the sender of this conversation entry.
* @returns {string}
*/
get sender() {
return this.conversationEntry.sender && this.conversationEntry.sender.role;
}
/**
* Returns the text content of the conversation entry.
* @returns {string}
*/
get textContent() {
try {
const entryPayload = JSON.parse( this.conversationEntry.entryPayload );
if (
entryPayload.abstractMessage &&
entryPayload.abstractMessage.staticContent
) {
const text = entryPayload.abstractMessage.staticContent.text;
return text;
}
return "";
} catch (e) {
// Handle JSON parsing error
console.error( e );
}
}
/**
* Returns the class name of the message bubble.
* @returns {string}
*/
get generateMessageBubbleClassname() {
if ( this.isSupportedSender() ) {
return `${ MESSAGE_CONTENT_CLASS } ${ this.sender }`;
} else {
throw new Error(
`Unsupported participant type passed in: ${ this.sender }`
);
}
}
/**
* True if the sender is a support participant type.
* @returns {Boolean}
*/
isSupportedSender() {
return PARTICIPANT_TYPES.some(
( participantType ) => this.sender === participantType,
);
}
}
CSS:
:host {
display: block;
}
.Agent,
.Chatbot {
float: left;
padding: 0.5em;
color: #FFFFFF;
background-color: #066AFE;
border-radius: 10px 10px 10px 0;
}
.EndUser {
padding: 0.5em;
color: white;
margin-left: auto;
background-color: #801818;
border-radius: 10px 10px 0 10px;
}
lightning-formatted-text {
font-size: 0.875em;
}
js-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>63.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightningSnapin__MessagingTextMessageBubble</target>
</targets>
</LightningComponentBundle>
Output:
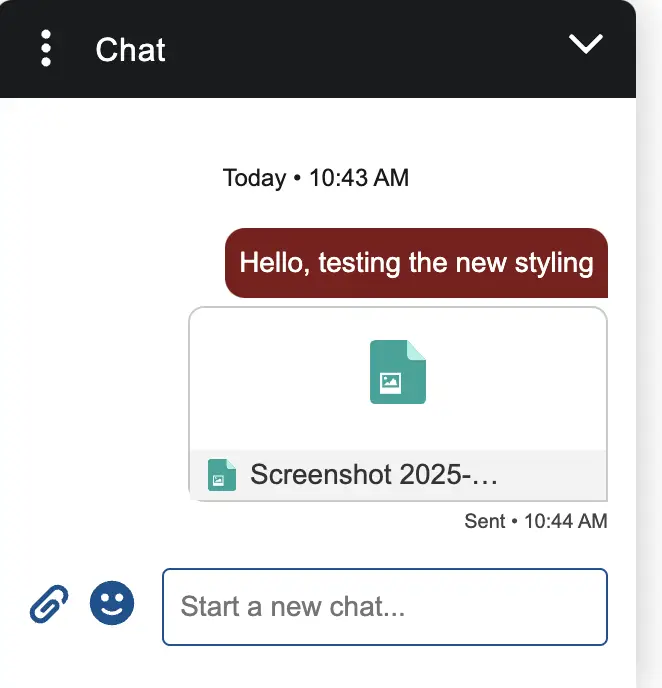