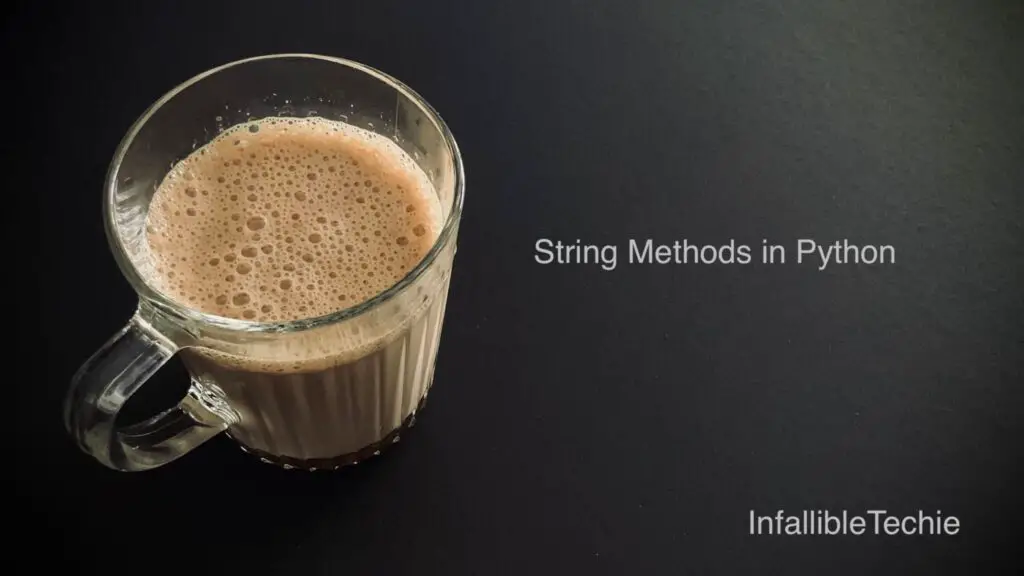
len():
len() is used to find the length of a string.
Sample Code:
strInfo = input(
'Enter a String: '
);
print(
'Length of ' +
strInfo +
' is',
len( strInfo )
);
Slicing String:
We can slice the string using the start and end indexes.
Sample Code:
strInfo = input(
'Enter a String: '
);
strStart = input(
'Enter Start Index: '
);
strEnd = input(
'Enter End Index: '
);
intStart = int( strStart );
intEnd = int ( strEnd );
print(
strInfo[ intStart : intEnd ]
);
lower():
lower() is used to convert it to lowercase.
Sample Code:
strInfo = input(
'Enter a String in Uppercase: '
);
print(
'Lowercase is',
strInfo.lower()
);
upper():
upper() is used to convert the string to uppercase.
Sample Code:
strInfo = input(
'Enter a String in Lowercase: '
);
print(
'Uppercase is',
strInfo.upper()
);
replace():
replace() is used to replace search string with replacement string.
Sample Code:
strInfo = 'Testing Python';
print(
'Testing replaced with Test is',
strInfo.replace( 'Testing', 'Test' )
);
lstrip():
Removes whitespace on the left/beginning.
rstrip():
Removes whitespace on the right/ending.
strip():
Removes whitespaces in the beginning and ending.
startswith():
Returns a boolean value whether the string starts with the specified string.
find():
Returns the position of specified String.
Sample Code:
strInfo = 'test # example';
print(
'Position of # is',
strInfo.find( '#' )
);