HTML:
<template>
<div class="slds-box slds-theme--default">
<lightning-record-edit-form record-id={recordId} object-api-name={objectAPIName} onsuccess={handleSuccess} onsubmit={handleSubmit}>
<lightning-messages>
</lightning-messages>
<lightning-input-address
address-label="Address"
street-label="Street"
city-label="City"
country-label="Country"
province-label="State/ Province"
postal-code-label="Zip/ Postal Code"
onchange={addressInputChange}
show-address-lookup>
</lightning-input-address>
<lightning-button class="slds-m-top_small" type="submit" label="Update Address">
</lightning-button>
</lightning-record-edit-form>
</div>
</template>
JavaScript:
import { LightningElement,api } from 'lwc';
import { ShowToastEvent } from 'lightning/platformShowToastEvent';
export default class AddressLwc extends LightningElement {
@api recordId;
@api objectAPIName;
strStreet;
strCity;
strState;
strCountry;
strPostalCode;
handleSuccess( event ) {
console.log( 'Updated Record Id is ', event.detail.id );
this.dispatchEvent(
new ShowToastEvent({
title: 'Successful Record Update',
message: 'Record Updated Surccessfully!!!',
variant: 'success'
})
);
}
handleSubmit( event ) {
let fields = event.detail.fields;
console.log( 'Fields are ' + JSON.stringify( fields ) );
event.preventDefault();
if ( this.objectAPIName === 'Account' ) {
fields.BillingStreet = this.strStreet;
fields.BillingCity = this.strCity;
fields.BillingState = this.strState;
fields.BillingCountry = this.strCountry;
fields.BillingPostalCode = this.strPostalCode;
} else if ( this.objectAPIName === 'Contact' ) {
fields.MailingStreet = this.strStreet;
fields.MailingCity = this.strCity;
fields.MailingState = this.strState;
fields.MailingCountry = this.strCountry;
fields.MailingPostalCode = this.strPostalCode;
}
this.template.querySelector( 'lightning-record-edit-form' ).submit( fields );
}
addressInputChange( event ) {
this.strStreet = event.target.street;
this.strCity = event.target.city;
this.strState = event.target.province;
this.strCountry = event.target.country;
this.strPostalCode = event.target.postalCode;
}
}
js-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>50.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__RecordPage</target>
</targets>
<targetConfigs>
<targetConfig targets="lightning__RecordPage">
<property name="objectAPIName" type="String" label="Object API Name"/>
</targetConfig>
</targetConfigs>
</LightningComponentBundle>
Output:
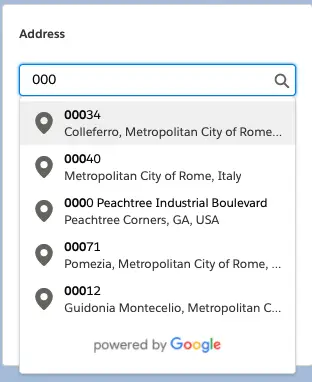