We can import the CSS from Static Resource using @salesforce/resourceUrl/StaticResourceAPIName and use it in the Lightning Web Component.
LightningCard.css:
.textCSS {
color: red;
}
.slds-card__header {
color: green;
font-weight: bold;
}
.slds-th__action {
color: blue;
justify-content: center !important;
}
.buttonCSS {
background: black;
color: white;
font-weight: bold;
font-size: 14px;
}
Static Resource:
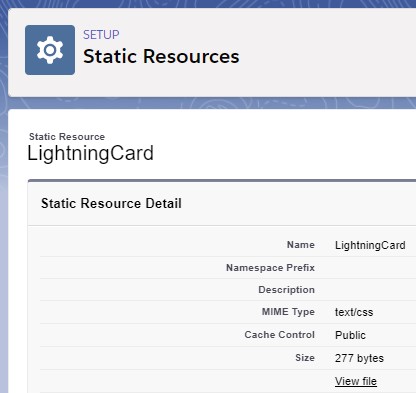
Apex Class:
public with sharing class LightningCardController {
@AuraEnabled( cacheable = true )
public static List < Case > fetchRecs( String strAccId ) {
List < Case > listRecs = new List < Case >();
String strSOQL = 'SELECT Id, Subject, Status, Origin FROM Case';
if ( String.isNotBlank( strAccId ) )
strSOQL += ' WHERE AccountId = \'' + strAccId + '\'';
strSOQL += ' LIMIT 3';
system.debug( 'SOQL is ' + strSOQL );
listRecs = Database.query( strSOQL );
return listRecs;
}
}
Lightning Web Component:
HTML:
<template>
<lightning-card title="Hello">
<button slot="actions" onclick={createNew} class="buttonCSS">New</button>
<p class="slds-p-horizontal_small">
<lightning-datatable key-field="Id"
data={listRecs}
columns={columns}
hide-checkbox-column="true"
class="textCSS">
</lightning-datatable>
</p>
<p slot="footer" class="slds-align_absolute-center">
<button onclick={navigateToCaseHome} class="buttonCSS">View All Cases</button>
</p>
</lightning-card>
<template if:true = {error}>
{error}>
</template>
</template>
JavaScript:
import { api, LightningElement, wire } from 'lwc';
import fetchRecs from '@salesforce/apex/LightningCardController.fetchRecs';
import { NavigationMixin } from 'lightning/navigation';
import { loadStyle } from 'lightning/platformResourceLoader';
import LightningCardCSS from '@salesforce/resourceUrl/LightningCard';
const columns = [
{ label: 'Subject', fieldName: 'Subject' },
{ label: 'Status', fieldName: 'Status' },
{ label: 'Case Origin', fieldName: 'Origin' }
];
export default class LightningCard extends NavigationMixin( LightningElement ) {
@api AccountId;
columns = columns;
listRecs;
error;
renderedCallback() {
Promise.all([
loadStyle( this, LightningCardCSS )
]).then(() => {
console.log( 'Files loaded' );
})
.catch(error => {
console.log( error.body.message );
});
}
@wire(fetchRecs, { strAccId: '$AccountId' })
wiredRecs( { error, data } ) {
if ( data ) {
console.log( 'Records are ' + JSON.stringify( data ) );
this.listRecs = data;
} else if ( error ) {
this.listRecs = null;
this.error = error;
}
}
createNew() {
this[NavigationMixin.Navigate]({
type: 'standard__objectPage',
attributes: {
objectApiName: 'Case',
actionName: 'new'
}
});
}
navigateToCaseHome() {
this[NavigationMixin.Navigate]({
type: 'standard__objectPage',
attributes: {
objectApiName: 'Case',
actionName: 'home'
}
});
}
}
meta.xml:
<?xml version=”1.0″ encoding=”UTF-8″?>
<LightningComponentBundle xmlns=”http://soap.sforce.com/2006/04/metadata”>
<apiVersion>49.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightningCommunity__Page</target>
<target>lightningCommunity__Default</target>
</targets>
<targetConfigs>
<targetConfig targets=”lightningCommunity__Default”>
<property name=”AccountId” type=”String”/>
</targetConfig>
</targetConfigs>
</LightningComponentBundle>
Output:
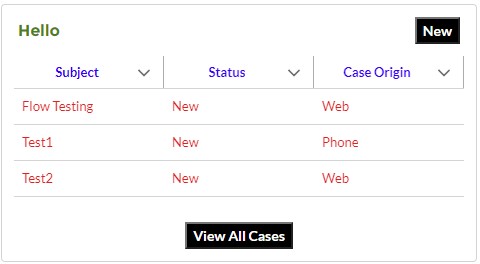