In Salesforce Lightning Web Component lightning-datatable, we can sort the records in JavaScript. We can avoid Server Calls(Apex) to sort the records using SOQL sorting.
Sample Code:
Apex Class:
public class AccountController {
@AuraEnabled( cacheable = true )
public static List< Account > fetchAccounts() {
return [
SELECT Id, Name, Industry,
Rating, AnnualRevenue, Type
FROM Account
LIMIT 10
];
}
}
LWC HTML:
<template>
<lightning-card>
<lightning-datatable
key-field="Id"
data={accountRecords}
columns={columns}
hide-checkbox-column="true"
show-row-number-column="true"
sorted-direction={sortDirection}
sorted-by={sortedBy}
onsort={onHandleSort}>
</lightning-datatable>
</lightning-card>
</template>
LWC JavaScript:
import { LightningElement, wire } from 'lwc';
import fetchAccounts from '@salesforce/apex/AccountController.fetchAccounts';
export default class sampleLightningWebComponent extends LightningElement {
accountRecords;
sortedBy;
sortDirection = 'asc';
columns = [
{ label: 'Name', fieldName: 'Name', sortable: true },
{ label: 'Industry', fieldName: 'Industry', sortable: true },
{ label: 'Rating', fieldName: 'Rating', sortable: true },
{ label: 'Annual Revenue', fieldName: 'AnnualRevenue', sortable: true },
{ label: 'Type', fieldName: 'Type', sortable: true }
];
@wire(fetchAccounts)
accountData( { error, data } ) {
if ( data ) {
console.log(
'Account Records are',
JSON.stringify( data )
);
this.accountRecords = data;
} else if ( error ) {
console.log(
JSON.stringify( error )
);
}
}
onHandleSort( event ) {
const { fieldName: columnName, sortDirection } = event.detail;
console.log(
'Column Name is',
columnName
);
console.log(
'sortDirection is',
sortDirection
);
this.sortDirection = sortDirection ;
let isReverse = this.sortDirection === 'asc' ? 1 : -1;
this.sortedBy = columnName;
this.accountRecords = JSON.parse( JSON.stringify( this.accountRecords ) ).sort( ( a, b ) => {
a = a[ columnName ] ? a[ columnName ] : '';
b = b[ columnName ] ? b[ columnName ] : '';
return a > b ? 1 * isReverse : -1 * isReverse;
} );
}
}
LWC meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>58.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__Tab</target>
</targets>
</LightningComponentBundle>
Output:
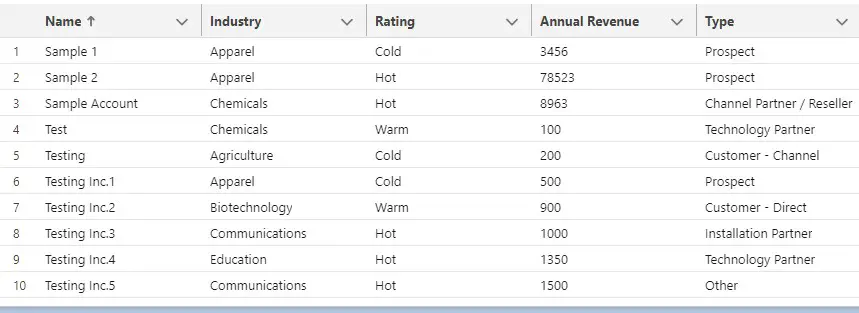
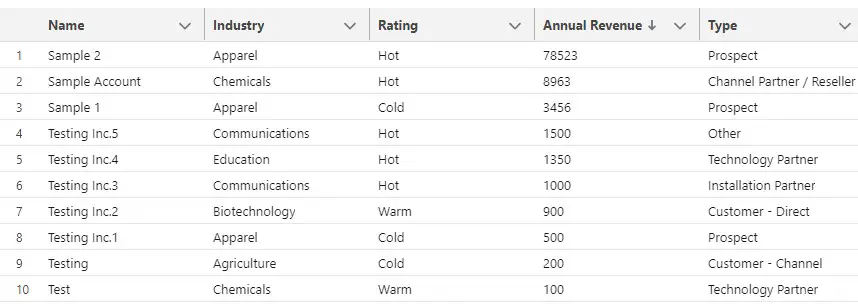