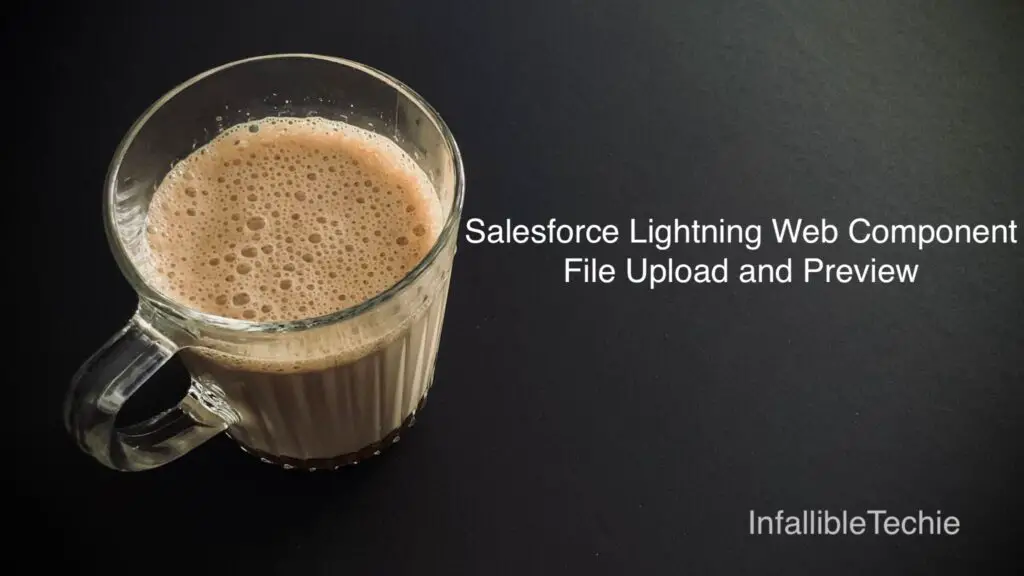
To preview the File in Salesforce Lightning Web Component, we need the Content Document Id and the Content Version Id.
Sample Lightning Web Component:
HTML:
<template>
<lightning-card>
<div class="slds-m-around_medium">
<template if:true={fileName}>
Preview Uploaded Image -
<a onclick={previewImage}>
{fileName}
</a>
<br/><br/>
<img src={imageURL}/>
</template>
<lightning-file-upload
label="Attach Image"
accept={acceptedFormats}
record-id={recordId}
onuploadfinished={handleUploadFinished}>
</lightning-file-upload>
</div>
</lightning-card>
</template>
JavaScript:
import { LightningElement, api } from 'lwc';
import { NavigationMixin } from 'lightning/navigation'
export default class AccountFileUpload extends NavigationMixin( LightningElement ) {
@api recordId;
fileName;
strContentDocId;
strContentVerId;
imageURL;
get acceptedFormats() {
return [
'.jpeg',
'.png'
];
}
handleUploadFinished( event ) {
let uploadedFilesList = event.detail.files;
let uploadedFile = uploadedFilesList[ 0 ];
console.log(
'uploadedFile is',
JSON.stringify(
uploadedFile
)
);
this.strContentDocId = uploadedFile.documentId;
this.fileName = uploadedFile.name;
this.strContentVerId = uploadedFile.contentVersionId;
this.imageURL = '/sfc/servlet.shepherd/version/'+
'renditionDownload?rendition=' +
'THUMB240BY180&versionId=' +
this.strContentVerId+
'&operationContext=CHATTER&contentId=' +
this.strContentDocId;
}
previewImage() {
this[ NavigationMixin.Navigate ] ( {
type: 'standard__namedPage',
attributes: {
pageName: 'filePreview'
},
state:{
selectedRecordId: this.strContentDocId
}
}, false );
}
}
js-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>59.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__RecordPage</target>
</targets>
</LightningComponentBundle>
Output:
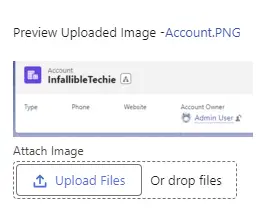