lightningsnapin/basePrechat can be used in Lightning Web Component LWC to validate customer input on the pre-chat before invoking the Chat in Salesforce Embedded Service Deployment.
Sample Lightning Web Component:
HTML:
<template>
<div style="height: 100%; overflow:scroll;">
<lightning-card title="Pre-Chat Form">
<template if:true={errorMessages}>
<div style="color:red;font-weight:bold;">
<lightning-formatted-rich-text
value={errorMessages}>
</lightning-formatted-rich-text>
</div>
</template>
<template
class="slds-m-around_medium"
for:each={fields}
for:item="field">
<div key={field.name}>
<lightning-input
key={field.name}
name={field.name}
label={field.label}
value={field.value}
max-length={field.maxLength}
required={field.required}>
</lightning-input>
</div>
</template>
</lightning-card>
<br/><br/>
<lightning-button
label="Start Chat"
title="Start Chat"
onclick={handleStartChat}
class="slds-m-left_x-small"
variant="brand">
</lightning-button>
</div>
</template>
JavaScript:
import BasePrechat from 'lightningsnapin/basePrechat';
import { api } from 'lwc';
export default class PreChatComponent extends BasePrechat {
@api prechatFields;
fields;
namelist;
errorMessages;
/**
* Set the button label and prepare the prechat fields to be shown in the form.
*/
connectedCallback() {
this.fields = this.prechatFields.map( field => {
const { label, name, value, required, maxLength } = field;
return { label, value, name, required, maxLength };
});
this.namelist = this.fields.map( field => field.name );
}
/**
* On clicking the 'Start Chatting' button, send a chat request.
*/
handleStartChat() {
this.template.querySelectorAll( "lightning-input" ).forEach( input => {
this.fields[ this.namelist.indexOf( input.name ) ].value = input.value;
});
if ( this.validateFields( this.fields ).valid ) {
let checkBool = true;
this.errorMessages = 'Please fix the following error(s):<br/>';
for ( let field of this.fields ) {
console.log( JSON.stringify( field ) );
console.log( 'Field Value is ' + field.value );
if ( !field.value ) {
checkBool = false;
this.errorMessages += 'Please fill ' + field.label + '<br/>';
}
if ( field.label === 'Phone' ) {
if ( field.value ) {
if (
field.value.match(
/\d/g
).length !=10
) {
checkBool = false;
this.errorMessages += 'Please enter 10 digit Phone Number<br/>';
}
}
}
if ( field.label === 'Email' ) {
console.log(
'Inside Email Validation'
);
if ( field.value ) {
let strEmailRegEx = /^[^\s@]+@[^\s@]+\.[^\s@]+$/;
if (
! strEmailRegEx.test(
field.value
)
) {
checkBool = false;
this.errorMessages += 'Please enter valid Email<br/>';
}
}
}
}
if ( checkBool === true ) {
console.log( 'Starting Chat' );
this.startChat( this.fields );
} else {
console.log(
'Error Message(s)',
this.errorMessages
);
}
} else {
console.log( 'Some error in initiating the Chat' );
}
}
}
js-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>59.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightningSnapin__PreChat</target>
</targets>
</LightningComponentBundle>
Pre-Chat Config in Embedded Service Deployment:
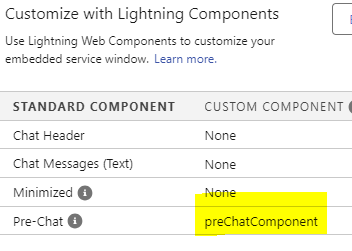
Output:
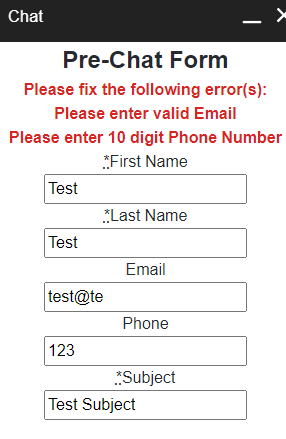