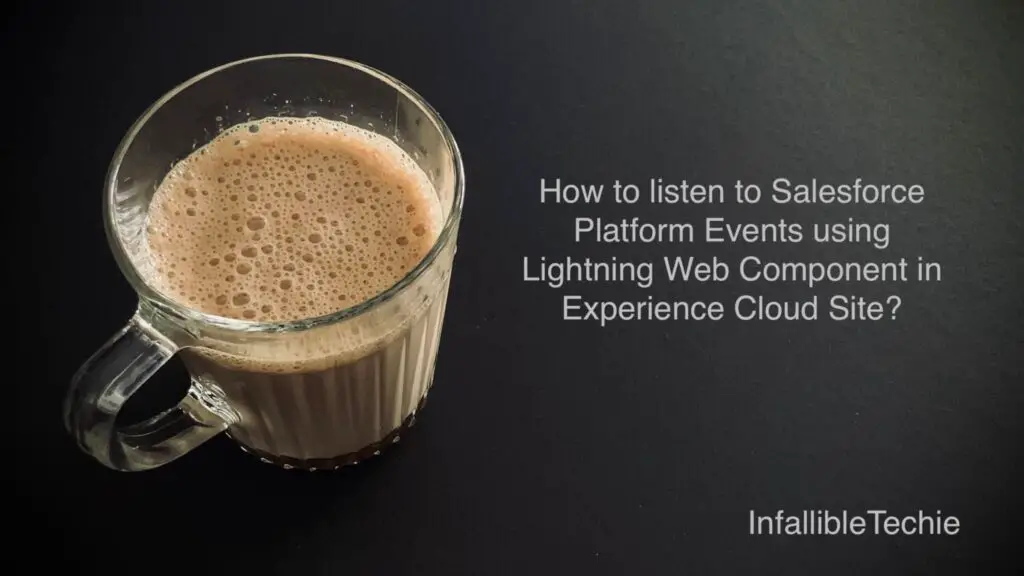
CometD can be used to listen to Salesforce Platform Events using Lightning Web Component in Experience Cloud Site.
1. Go to https://download.cometd.org/ and download the CometD. I used cometd-7.0.9.
2. Extract the downloaded CometD file. Open /cometd-7.0.9/cometd-javascript/common/src/main/webapp/js/cometd and upload the cometd.js to a Static Resource.
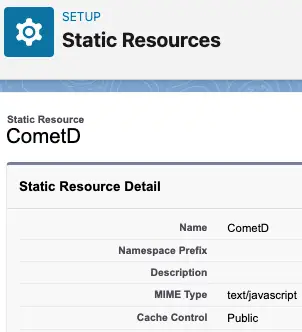
3. Create a Platform Event.
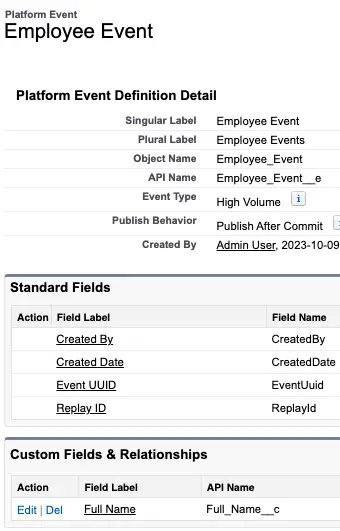
4. Give Read access to the Experience Cloud Site User Profile for the Platform Event.
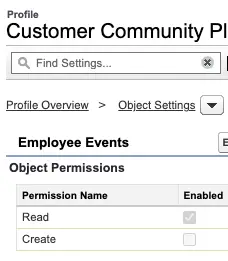
5. Create the following Apex class. This Apex class will be called from the Lightning Web Component to get the current User’s Session Id to listen to the Platform Events.
public class PlatformEventListenerController {
@AuraEnabled( cacheable=true )
public static String fetchSessionId() {
return UserInfo.getSessionId();
}
}
6. Give Read access to the Experience Cloud Site User Profile for the Apex Class.
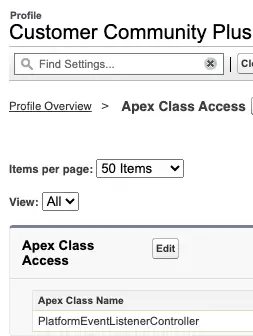
7. Create the following Lightning Web Component.
HTML:
<template>
</template>
JavaScript:
import { LightningElement, wire } from 'lwc';
import { loadScript } from "lightning/platformResourceLoader";
import CometD from "@salesforce/resourceUrl/CometD";
import fetchSessionId from '@salesforce/apex/PlatformEventListenerController.fetchSessionId';
export default class PlatformEventListener extends LightningElement {
sessionId;
@wire( fetchSessionId )
wiredSessionId(
{ error, data }
) {
if ( data ) {
this.sessionId = data;
this.error = undefined;
loadScript( this, CometD )
.then( () => {
let cometDLib = new window.org.cometd.CometD();
cometDLib.configure( {
url: window.location.protocol +
'//' + window.location.hostname
+ '/cometd/58.0/',
requestHeaders: {
Authorization: 'OAuth ' +
this.sessionId
},
appendMessageTypeToURL : false,
logLevel: 'debug'
} );
cometDLib.websocketEnabled = false;
cometDLib.handshake( function( status ) {
console.log(
'Status is',
JSON.stringify(
status
)
);
if ( status.successful ) {
cometDLib.subscribe(
'/event/Employee_Event__e',
function( message
) {
console.log(
JSON.stringify(
message
)
);
});
} else {
console.error(
'Error in handshaking:',
JSON.stringify(
status
)
);
}
} );
} );
} else if ( error ) {
console.log(
'Error occurred in loading script'
);
console.log(
JSON.stringify(
error
)
);
this.sessionId = undefined;
}
}
}
js-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>58.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightningCommunity__Page</target>
</targets>
</LightningComponentBundle>
8. Add the Lightning Web Component to the Experience Cloud Site to test.
9. Use the following Sample Apex Code to publish couple of Platform Events.
List < Employee_Event__e > listEvents = new List < Employee_Event__e >();
listEvents.add(
new Employee_Event__e(
Full_Name__c = 'Test 1'
)
);
listEvents.add(
new Employee_Event__e(
Full_Name__c = 'Test 2'
)
);
List < Database.SaveResult > results = EventBus.publish(
listEvents
);
for ( Database.SaveResult sr : results ) {
if ( sr.isSuccess() ) {
System.debug(
'Event successfully published event.'
);
} else {
for(Database.Error err : sr.getErrors()) {
System.debug(
'Error code: ' +
err.getStatusCode()
);
System.debug(
'Error message: ' +
err.getMessage()
);
}
}
}
Output:
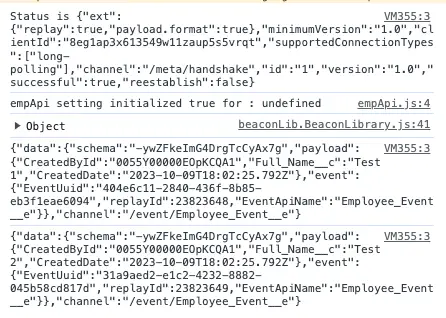