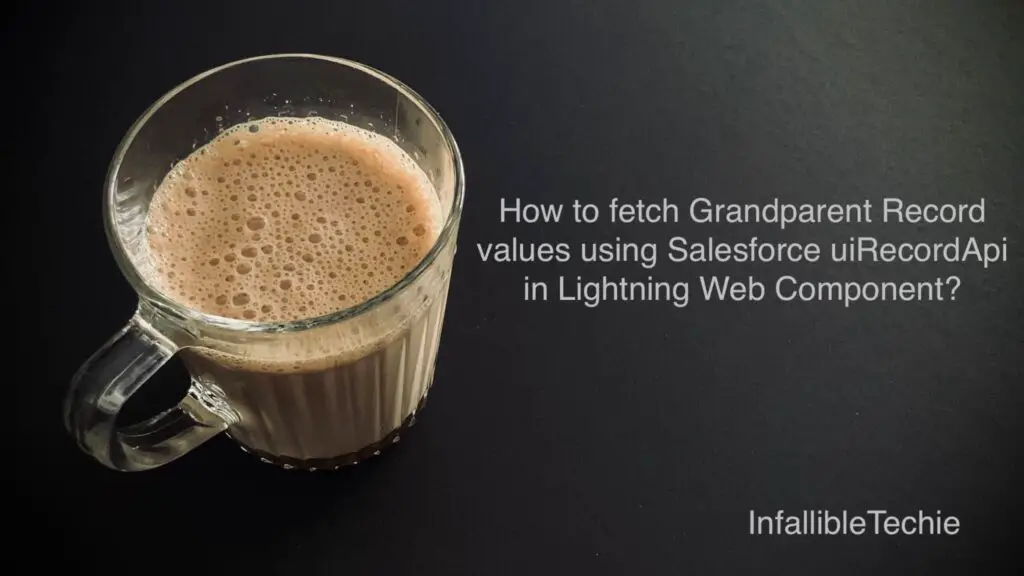
In Salesforce SOQL, we use dot notation to get the parent record information. Similarly, we can do the same in the Lightning Web Component uiRecordApi.
Sample Lightning Web Component:
HTML:
<template>
<div
class="slds-notify slds-notify_alert slds-alert_error"
role="alert">
<h2>
{accountName}
</h2>
</div>
</template>
JavaScript:
import { LightningElement, api, wire } from 'lwc';
import { getRecord, getFieldValue } from 'lightning/uiRecordApi';
import ACCOUNT_NAME from "@salesforce/schema/LiveChatTranscript.Contact.Account.Name";
import { ShowToastEvent } from 'lightning/platformShowToastEvent';
export default class AccountDetailsForChat extends LightningElement {
accountName;
@api recordId;
@wire( getRecord, {
recordId: '$recordId',
fields: [ ACCOUNT_NAME ]
} )
wiredRecord({ error, data }) {
if ( error ) {
console.log(
'Inside error block'
);
let message = 'Unknown error';
if ( Array.isArray( error.body ) ) {
message = error.body.map(e => e.message).join(', ');
} else if ( typeof error.body.message === 'string' ) {
message = error.body.message;
}
this.dispatchEvent(
new ShowToastEvent( {
title: 'Some error occurred',
message,
variant: 'error',
} ),
);
} else if ( data ) {
console.log(
'Account Name Value is',
getFieldValue( data, ACCOUNT_NAME )
);
this.accountName = getFieldValue( data, ACCOUNT_NAME );
}
}
}
js-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>58.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__RecordPage</target>
</targets>
</LightningComponentBundle>
Output:
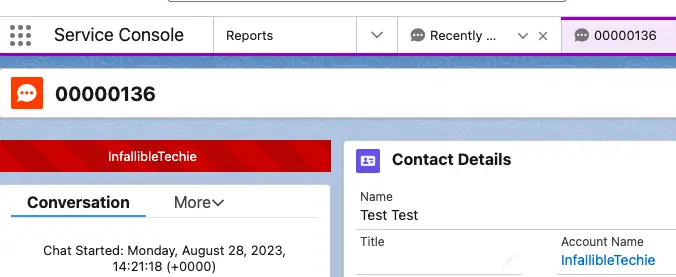