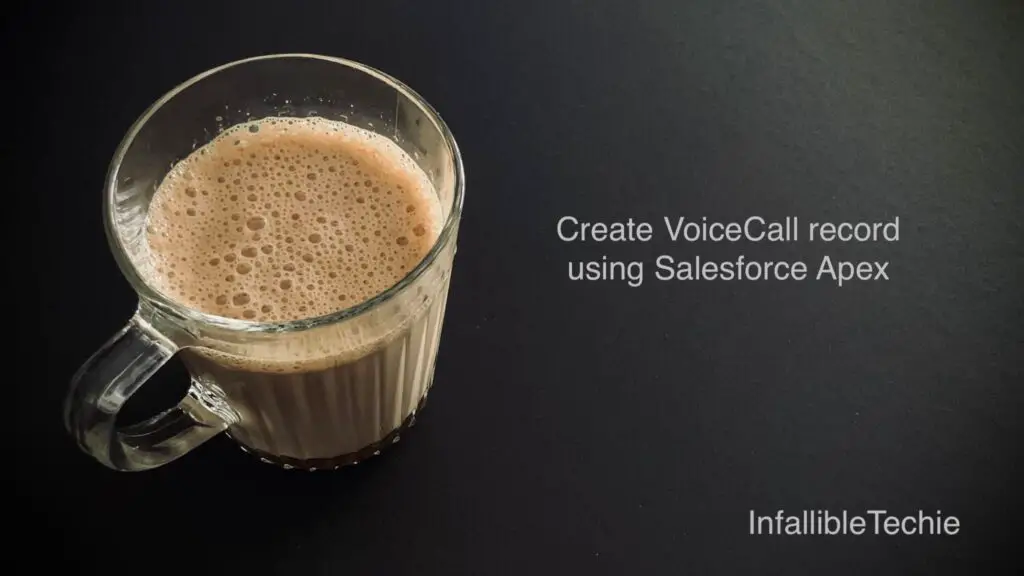
1. Use the following SOQL to get the CallCenterId.
SELECT Id, Name
FROM CallCenter
2. Use the following Apex code to create the VoiceCall record.
Sample Apex Code:
insert new VoiceCall(
CallCenterId = '<Id of Contact Center>',
VendorType = 'ContactCenter',
CallType = 'Inbound',
FromPhoneNumber = '+19999999999',
ToPhoneNumber = '+19999999999',
CallStartDateTime = System.now().addMinutes( - 3 ),
CallEndDateTime = System.now(),
CallDisposition = 'completed'
);
Sample Apex Code:
public with sharing class VoiceUtility {
public static void createVoiceCall(
String callCenterName
) {
List < CallCenter > listCallCenters = [
SELECT Id
FROM CallCenter
WHERE Name =: callCenterName
];
CallCenter objCC =
listCallCenters.size() > 0 ?
listCallCenters.get( 0 ) :
null;
insert new VoiceCall(
CallCenterId = objCC != null ? objCC.Id : null,
VendorType = 'ContactCenter',
CallType = 'Inbound',
FromPhoneNumber = '+19999999999',
ToPhoneNumber = '+19999999999',
CallStartDateTime = System.now().addMinutes( - 3 ),
CallEndDateTime = System.now(),
CallDisposition = 'completed'
);
}
}
Sample Apex Code to execute the Utility Class and Method:
VoiceUtility.createVoiceCall(
'InfallibleTechVoice'
);
Test Class for VoiceUtility:
@isTest
public class VoiceUtilityTest {
static testMethod void testGet() {
VoiceUtility.createVoiceCall(
'Testing'
);
}
}