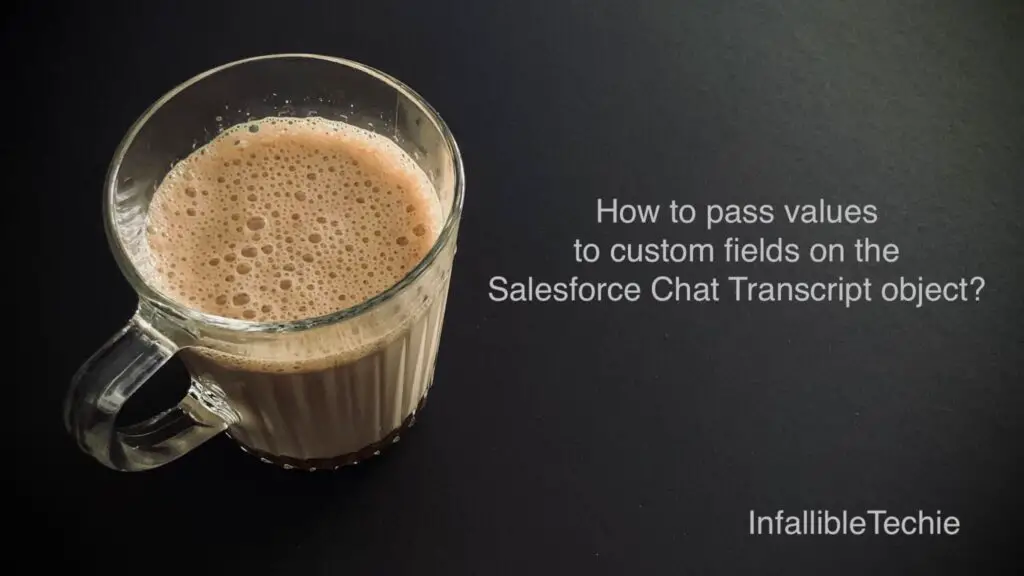
Use the following steps to pass values to custom fields on the Salesforce Chat Transcript object(LiveChatTranscript).
1. Create the Custom Fields on the Salesforce Chat Transcript object(LiveChatTranscript).
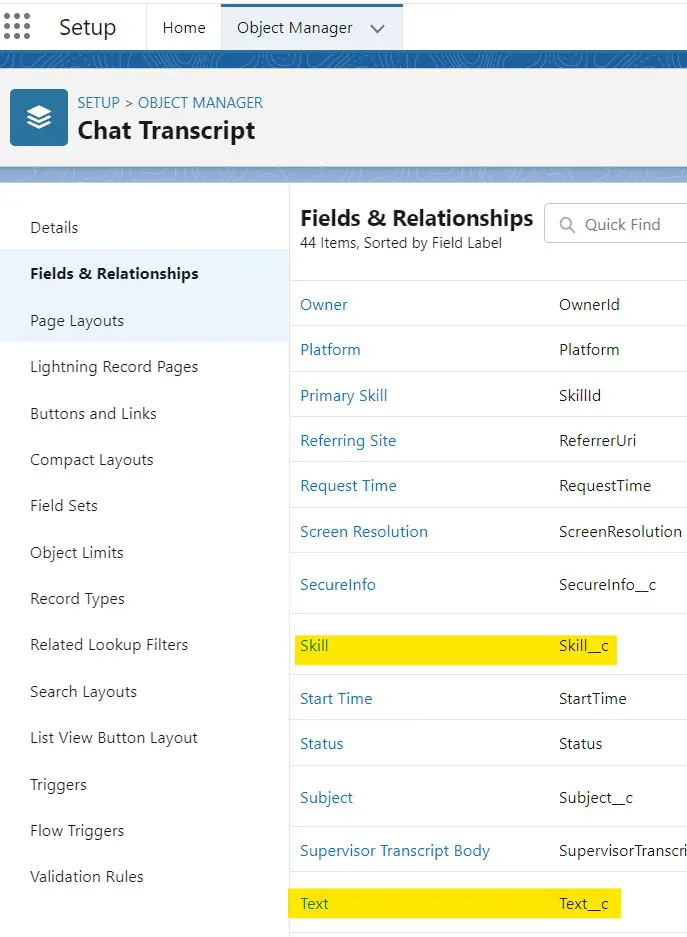
2. Add the following code within your Chat Code Snippet.
embedded_svc.settings.extraPrechatFormDetails = [
{
"label":"Text",
"transcriptFields": [ "Text__c" ]
},
{
"label":"Skill",
"transcriptFields": [ "Skill__c" ]
}
];
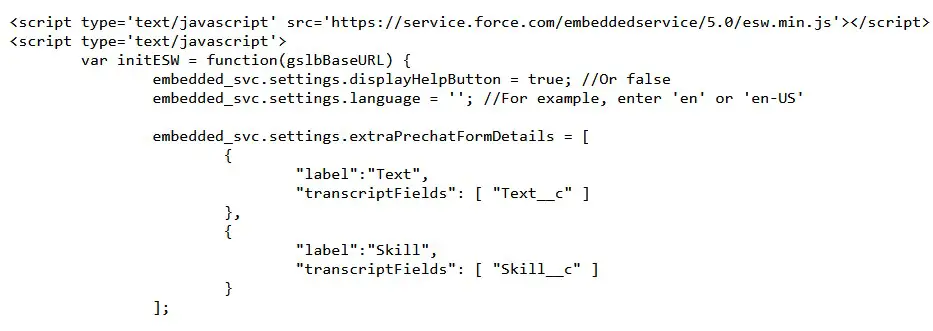
3. Add the following code in the website where your Chat Code Snippet is hosted. I have added it just within the script tags of the Chat Code Snippet.
document.addEventListener(
"setCustomField",
function( event ) {
console.log(
'Inside the Custom Field Listener'
);
console.log(
'Passed Text value is',
event.detail.text
);
console.log(
'Passed Skill value is',
event.detail.skill
);
embedded_svc.settings.extraPrechatFormDetails[ 0 ].value = event.detail.text;
embedded_svc.settings.extraPrechatFormDetails[ 1 ].value = event.detail.skill;
event.detail.callback();
},
false
);
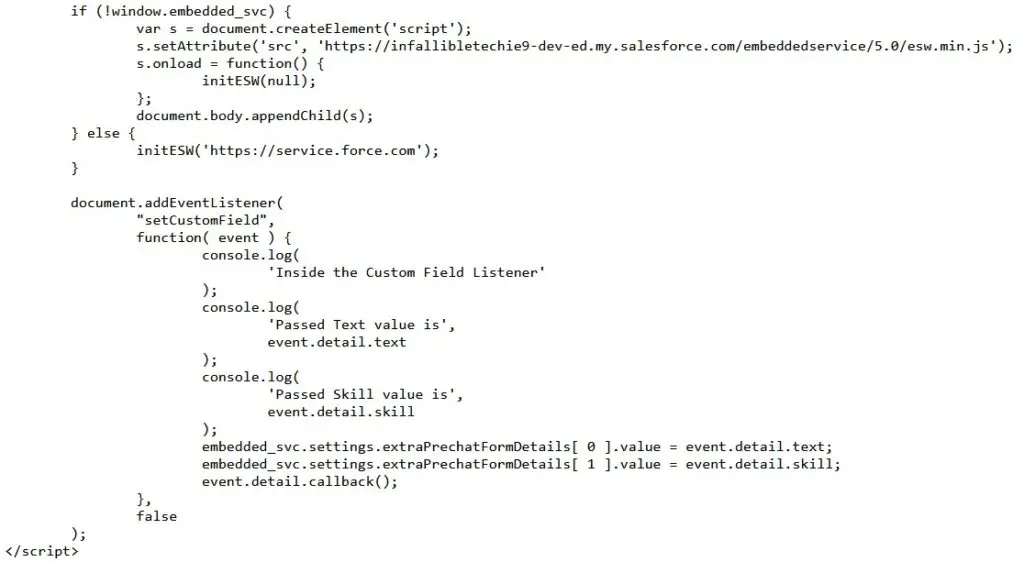
4. Create the following Lightning Aura Component for Pre-Chat form. We can use this Pre-Chat Lightning Aura Component to pass values to the extraPrechatFormDetails using custom event along with startChat as the callback function to initiate the chat.
Lightning Aura Component:
Component:
<aura:component implements="lightningsnapin:prechatUI">
<lightningsnapin:prechatAPI aura:id="prechatAPI"/>
<aura:handler name="init" value="{!this}" action="{!c.onInit}" />
<aura:attribute name="errorBool" type="Boolean" default="false"/>
<aura:attribute name="prechatFieldComponents" type="List"/>
<h2>Prechat form</h2>
<div>
<div>
<table cellspacing="10" cellpadding="10" width="100%">
<aura:iteration items="{!v.prechatFieldComponents}" var="field">
<tr>{!field}<br/></tr>
</aura:iteration>
</table>
<br/>
<aura:if isTrue="{!v.errorBool}">
<div class="errorMessage">
Please fill in all the required fields!!!
</div>
</aura:if>
<ui:button aura:id="startButton" label="Start Chat" press="{!c.handleStartButtonClick}"/>
</div>
</div>
</aura:component>
JavaScript:
({
onInit : function( component, event, helper ) {
let prechatFields = component.find(
"prechatAPI"
).getPrechatFields();
let prechatFieldComponentsArray = helper.getPrechatFieldAttributesArray(
prechatFields
);
$A.createComponents(
prechatFieldComponentsArray,
function( components, status, errorMessage ) {
if ( status === "SUCCESS" ) {
component.set(
"v.prechatFieldComponents",
components
);
}
}
);
},
handleStartButtonClick: function( component, event, helper ) {
helper.onStartButtonClick(
component
);
}
})
Helper:
({
fieldLabelToName: {
"First Name": "FirstName",
"Last Name": "LastName",
"Email": "Email",
"Subject": "Subject"
},
getPrechatFieldAttributesArray: function( prechatFields ) {
let prechatFieldsInfoArray = [];
prechatFields.forEach( function( field ) {
let componentName = ( field.type === "inputSplitName" ) ? "inputText" : field.type;
let componentInfoArray = [ "ui:" + componentName ];
let attributes = {
"aura:id": "prechatField",
required: field.required,
label: field.label,
disabled: field.readOnly,
maxlength: field.maxLength,
class: field.className,
value: field.value
};
if ( field.type === "inputSelect" && field.picklistOptions ) {
attributes.options = field.picklistOptions;
}
componentInfoArray.push(
attributes
);
prechatFieldsInfoArray.push(
componentInfoArray
);
});
return prechatFieldsInfoArray;
},
onStartButtonClick: function( component ) {
let prechatFieldComponents = component.find(
"prechatField"
);
let fields;
fields = this.createFieldsArray(
prechatFieldComponents
);
if ( component.find( "prechatAPI").validateFields( fields ).valid ) {
if (
fields[ 0 ].value &&
fields[ 1 ].value &&
fields[ 2 ].value &&
fields[ 3 ].value
) {
let event = new CustomEvent(
"setCustomField",
{
detail: {
callback: component.find(
"prechatAPI"
).startChat.bind(
this, fields
),
text : "Testing",
skill: "Service"
}
}
);
document.dispatchEvent(
event
);
} else {
component.set(
"v.errorBool",
"true"
);
}
} else {
console.warn(
"Prechat fields did not pass validation!"
);
}
},
createFieldsArray: function( fields ) {
if( fields.length ) {
return fields.map( function( fieldCmp ) {
return {
label: fieldCmp.get( "v.label" ),
value: fieldCmp.get( "v.value" ),
name: this.fieldLabelToName[ fieldCmp.get( "v.label" ) ]
};
}.bind( this ) );
} else {
return [];
}
}
})
5. Go to Chat Setting in the Embedded Service Deployment. Use the above Lightning Aura Component as the Pre-Chat in the “Customize with Lightning Components” section.
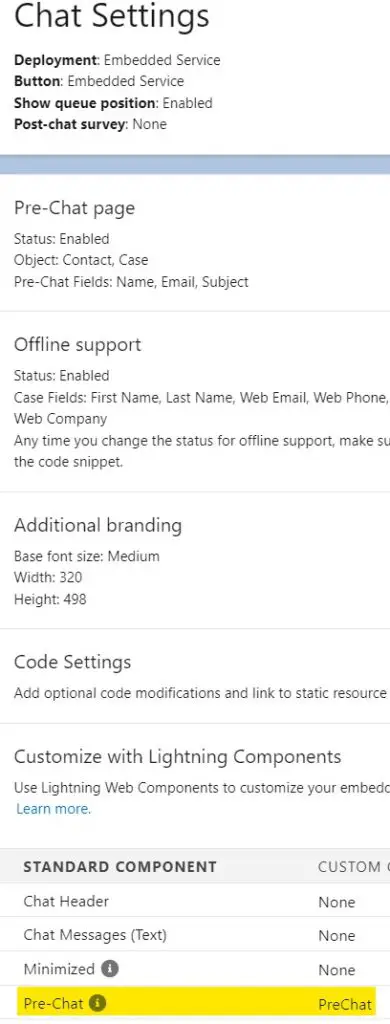
Output:
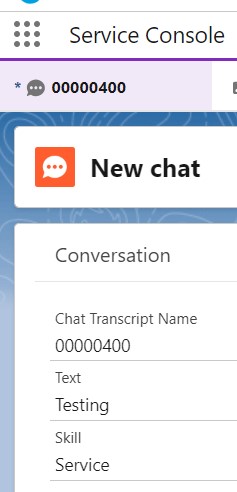
Reference Article:
https://help.salesforce.com/s/articleView?id=000380580&type=1