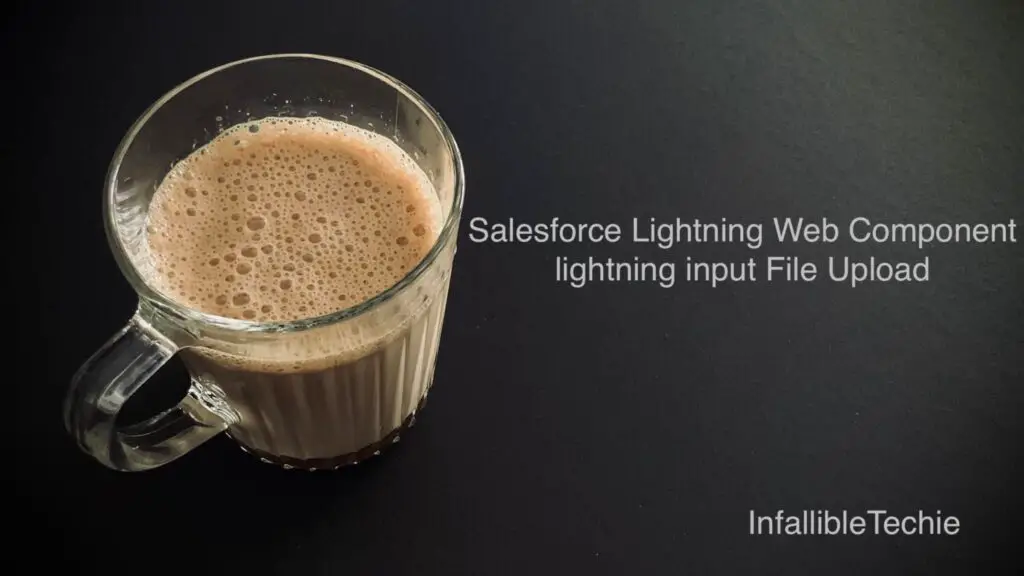
Using Salesforce Apex and FileReader() and encodeURIComponent() from JavaScript, we can easily upload a file using Lightning Web Component lightning:input of type file.
Sample Code:
Apex Class:
public class FileUploadController {
@AuraEnabled
public static String uploadFile(
String filename, String base64Content
) {
ContentVersion objContentVersion = new ContentVersion();
base64Content = EncodingUtil.urlDecode(
base64Content,
'UTF-8'
);
objContentVersion.VersionData = EncodingUtil.base64Decode(
base64Content
);
objContentVersion.Title = filename;
objContentVersion.PathOnClient = filename;
try {
insert objContentVersion;
} catch( DMLException e ) {
System.debug(
'Error while uploading the file ' +
e.getMessage()
);
return e.getMessage();
}
ContentDocumentLink objContentDocumentLink = new ContentDocumentLink();
objContentDocumentLink.ContentDocumentId = [
SELECT ContentDocumentId
FROM ContentVersion
WHERE Id =: objContentVersion.Id
].ContentDocumentId;
objContentDocumentLink.ShareType = 'V';
try {
insert objContentDocumentLink;
} catch( DMLException e ) {
System.debug(
'Error while uploading the file ' +
e.getMessage()
);
return e.getMessage();
}
return 'File Uploaded Successfully';
}
}
Lightning Web Component:
HTML:
<template>
<lightning-card title="File/Document/Attachment Upload">
<lightning-input
type="file"
onchange={handleFileUpload}>
</lightning-input>
{fileName}
<p slot="footer">
<lightning-button
label="Upload"
variant="brand"
onclick={submitFile}>
</lightning-button>
</p>
</lightning-card>
</template>
JavaScript:
import { LightningElement } from 'lwc';
import uploadFile from '@salesforce/apex/FileUploadController.uploadFile';
import { ShowToastEvent } from 'lightning/platformShowToastEvent';
export default class sampleLightningWebComponent extends LightningElement {
fileName;
fileInformation;
handleFileUpload( event ) {
let selectedFile = event.target.files[ 0 ];
this.fileName = selectedFile.name;
let reader = new FileReader();
reader.onload = () => {
let fileContent = reader.result.split(
','
)[ 1 ];
this.fileInformation = {
'fileName': selectedFile.name,
'fileContent': fileContent
}
}
reader.readAsDataURL(
selectedFile
);
}
submitFile() {
let fileName = this.fileInformation.fileName;
let fileContent = this.fileInformation.fileContent;
console.log(
'File Name is',
fileName
);
uploadFile( {
filename: fileName,
base64Content: encodeURIComponent(
fileContent
)
} ).then( result => {
this.fileName = null;
const toastEvent = new ShowToastEvent( {
title: 'File Uploaded',
message: 'File Uploaded Successfully!!!',
variant:"success"
} )
this.dispatchEvent( toastEvent );
} );
}
}
js-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>58.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__Tab</target>
</targets>
</LightningComponentBundle>
Output:
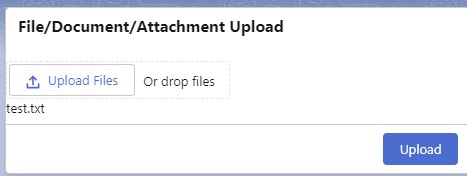
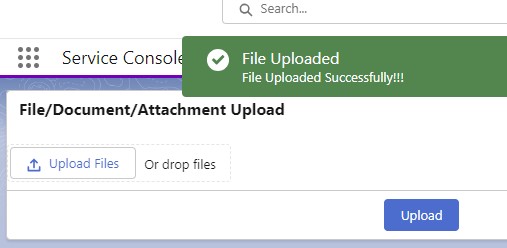