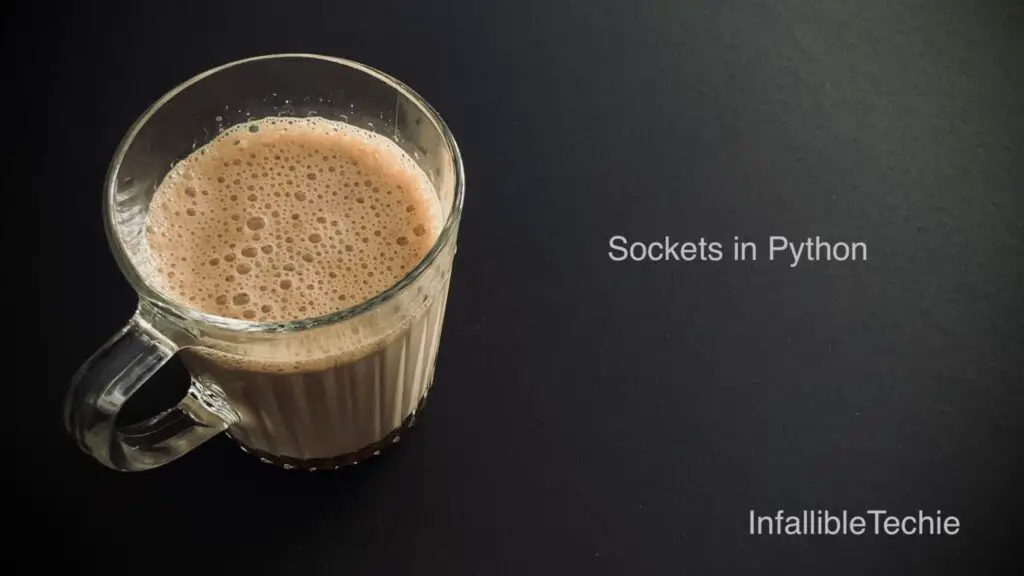
Python programming has built-in libraries for TCP Sockets. So, it makes life easier.
Syntax:
import socket
objSocket = socket.socket(
socket.AF_INET,
socket.SOCK_STREAM
);
encode() method can be used to encode when the request is made using send() method.
decode() method can be used to decode after receiving the response.
gethostbyname() will return the IP address of the host.
Sample Code:
import socket
strIP = socket.gethostbyname( 'www.infallibletechie.com' );
print(
'IP is',
strIP
);
gethostname() will return the hostname.
Sample Client Server Python Program:
sampleserver.py:
import socket
objServer = socket.socket(
socket.AF_INET,
socket.SOCK_STREAM
);
host = socket.gethostname();
objServer.bind( ( host, 8080 ) );
objServer.listen( 1 );
while True:
connection, address = objServer.accept();
strClientResponse = '';
while True:
data = connection.recv(4096);
if not data: break;
strClientResponse += data.decode();
print ( strClientResponse );
connection.send(
"Thank you from sever".encode()
);
connection.close();
print ( 'Client Connection Closed' );
sampleclient.py:
import socket
objClient = socket.socket(
socket.AF_INET,
socket.SOCK_STREAM
);
host = socket.gethostname();
objClient.connect( ( host, 8080 ) );
objClient.send( "Sample Client".encode() );
strServerResponse = objClient.recv( 1024 );
objClient.close();
print (
'Response from Server is',
strServerResponse.decode()
);
