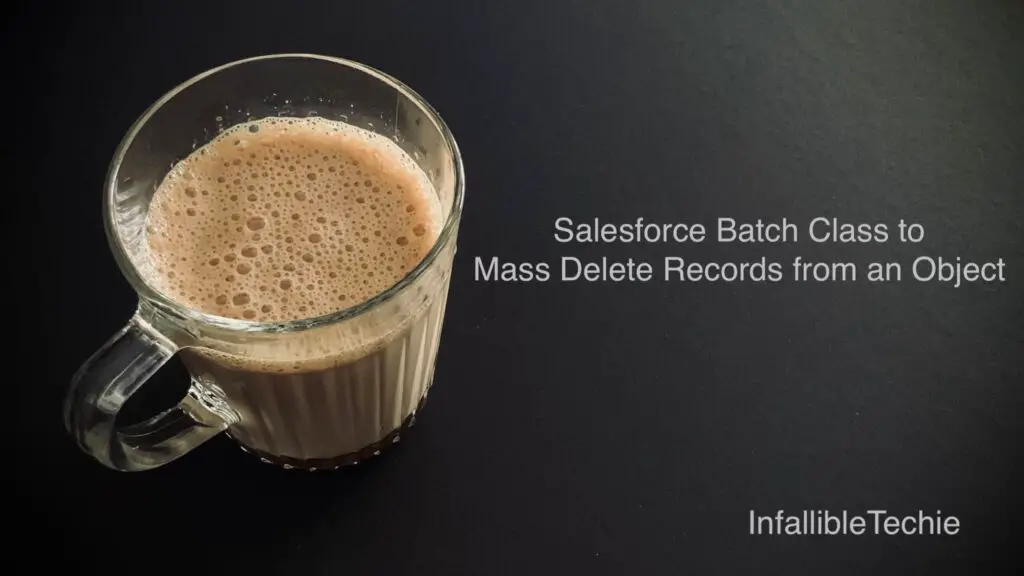
Salesforce Batch Apex can be used to mass or bulk delete records from objects in batches which will avoid hitting DML Governor Limit.
Check the following sample Batch Apex class to mass or bulk delete records from objects.
Sample Batch Apex for Mass Deleting Records:
global class MassDeleteRecords Implements Database.Batchable <sObject> {
String strObjectName;
Integer intLimit;
global MassDeleteRecords( String strObjectName, Integer intLimit ) {
this.strObjectName = strObjectName;
this.intLimit = intLimit;
}
global Database.queryLocator start(Database.BatchableContext bc) {
String SOQL = 'SELECT Id FROM ' + strObjectName + ' LIMIT ' + intLimit;
return Database.getQueryLocator( SOQL );
}
global void execute( Database.BatchableContext bc, List < sObject > listRecords ) {
delete listRecords;
}
global void finish( Database.BatchableContext bc ) {
}
}
Test Class for the Batch Apex for Mass Deleting Records:
@isTest
private class MassDeleteRecordsTest {
static testMethod void testMassDel() {
Account acc = new Account(
Name = 'Test Acc'
);
insert acc;
Test.startTest();
MassDeleteRecords objMDR = new MassDeleteRecords( 'Account', 5 );
Database.executeBatch( objMDR );
Test.stopTest();
Assert.areEqual( 0, [ SELECT COUNT() FROM Account ] );
}
}
To run the batch job for Opportunity records deletion, use the following code.
MassDeleteRecords objMDR = new MassDeleteRecords( 'Opportunity' );
Database.executeBatch( objMDR );