Sample Code:
Lightning Web Component:
HTML:
<template>
<lightning-card title="LdsCreateRecord" icon-name="standard:record">
<div class="slds-m-around_medium">
<lightning-input label="Account Name" onchange={handleNameChange} class="slds-m-bottom_x-small"></lightning-input>
<lightning-input label="Contact First Name" onchange={handleFirstNameChange} class="slds-m-bottom_x-small"></lightning-input>
<lightning-input label="Contact Last Name" onchange={handleLastNameChange} class="slds-m-bottom_x-small"></lightning-input>
<lightning-button label="Create Account and Contact" variant="brand" onclick={createRecords}></lightning-button>
</div>
</lightning-card>
</template>
JavaScript:
import { LightningElement } from 'lwc';
import { createRecord } from 'lightning/uiRecordApi';
import { ShowToastEvent } from 'lightning/platformShowToastEvent';
import ACCOUNT_OBJECT from '@salesforce/schema/Account';
import ACCOUNT_NAME_FIELD from '@salesforce/schema/Account.Name';
import CONTACT_OBJECT from '@salesforce/schema/Contact';
import CONTACT_ACCOUNT_Id_FIELD from '@salesforce/schema/Contact.AccountId';
import CONTACT_FIRST_NAME_FIELD from '@salesforce/schema/Contact.FirstName';
import CONTACT_LAST_NAME_FIELD from '@salesforce/schema/Contact.LastName';
export default class SampleLWC extends LightningElement {
accountName = '';
contactFirstName = '';
contactLastName = '';
handleNameChange( event ) {
this.accountName = event.target.value;
console.log( 'accountName is', this.accountName );
}
handleFirstNameChange( event ) {
this.contactFirstName = event.target.value;
console.log( 'contactFirstName is', this.contactFirstName );
}
handleLastNameChange( event ) {
this.contactLastName = event.target.value;
console.log( 'contactLastName is', this.contactLastName );
}
createRecords() {
let fields = {};
fields[ ACCOUNT_NAME_FIELD.fieldApiName ] = this.accountName;
console.log( 'accountFields is', JSON.stringify( fields ) );
const recordAccountInput = { apiName: ACCOUNT_OBJECT.objectApiName, fields };
createRecord( recordAccountInput )
.then( account => {
fields = {};
fields[ CONTACT_ACCOUNT_Id_FIELD.fieldApiName ] = account.id;
fields[ CONTACT_FIRST_NAME_FIELD.fieldApiName ] = this.contactFirstName;
fields[ CONTACT_LAST_NAME_FIELD.fieldApiName ] = this.contactLastName;
console.log( 'Contact Fields are', JSON.stringify( fields ) );
const recordContactInput = { apiName: CONTACT_OBJECT.objectApiName, fields };
createRecord( recordContactInput )
.then( contact => {
console.log( 'Contact Id is', contact.id );
this.dispatchEvent(
new ShowToastEvent( {
title: 'Success',
message: 'Account and Contact records created successfully!',
variant: 'success',
} ),
);
})
.catch( error => {
console.log( 'Error while creating Contact', JSON.stringify( error ) );
this.dispatchEvent(
new ShowToastEvent( {
title: 'Error creating Contact record',
message: error.body.message,
variant: 'error',
} ),
);
} );
})
.catch( error => {
console.log( 'Error while creating Account', JSON.stringify( error ) );
this.dispatchEvent(
new ShowToastEvent( {
title: 'Error creating Account record',
message: Object.keys( error ).length === 0 ? 'Some error occured while creating Account Record' : error.body.message,
variant: 'error',
} ),
);
} );
}
}
JS-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>54.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__Tab</target>
</targets>
</LightningComponentBundle>
Output:
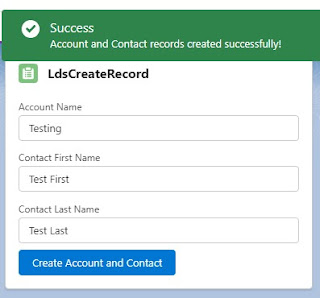
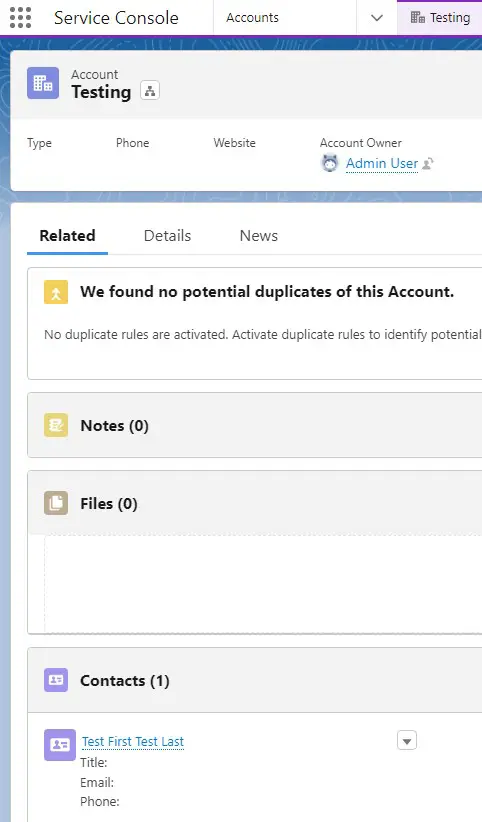