Sample code:
HTML:
<template>
<lightning-card>
<lightning-combobox
name="filter"
label="Industry"
value={selectedValue}
options={options}
onchange={handlePicklistChange}
placeholder="Select Industry"
read-only={readOnlyBool}>
</lightning-combobox>
<p slot="footer">
<lightning-button onclick={enablePicklist} label="Enable"></lightning-button>
<lightning-button onclick={disablePicklist} label="Disable"></lightning-button>
</p>
</lightning-card>
</template>
JavaScript:
import { LightningElement, wire } from 'lwc';
import INDUSTRY_FIELD from '@salesforce/schema/Account.Industry';
import { getPicklistValues, getObjectInfo } from 'lightning/uiObjectInfoApi';
import ACCOUNT_OBJECT from '@salesforce/schema/Account';
export default class LightningComboBox extends LightningElement {
options = [];
picklistOptions = [];
selectedValue;
readOnlyBool = false;
@wire( getObjectInfo, { objectApiName: ACCOUNT_OBJECT } )
objectInfo;
@wire( getPicklistValues, { recordTypeId: '$objectInfo.data.defaultRecordTypeId', fieldApiName: INDUSTRY_FIELD } )
wiredData( { error, data } ) {
console.log( 'Inside Get Picklist Values' );
if ( data ) {
console.log( 'Data received from Picklist Field ' + JSON.stringify( data.values ) );
this.options = data.values.map( objPL => {
return {
label: `${objPL.label}`,
value: `${objPL.value}`
};
});
console.log( 'Options are ' + JSON.stringify( this.options ) );
} else if ( error ) {
console.error( JSON.stringify( error ) );
}
}
handlePicklistChange( event ) {
console.log( 'New Value selected is ' + JSON.stringify( event.detail.value ) );
}
enablePicklist() {
this.readOnlyBool = false;
}
disablePicklist() {
this.readOnlyBool = true;
}
}
JS-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>52.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__Tab</target>
</targets>
</LightningComponentBundle>
Enabled Lightning Combo box
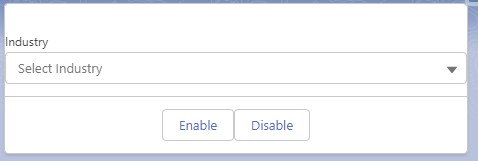
Disabled Lightning Combo box
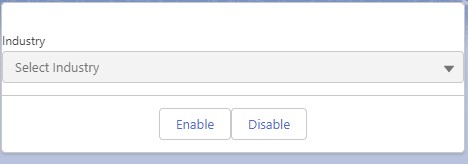