lightning/uiRelatedListApi can be used to fetch the related records and display using Lightning Web Component. Since we use lightning/uiRelatedListApi, apex code is not required to fetch the related records. Check the sample code below:
Sample Code:
HTML:
<template>
<lightning-card>
<div class="slds-m-around_medium">
No of Contacts is {recordCount}<br/><br/>
<lightning-datatable
key-field="Id"
data={records}
columns={columns}
hide-checkbox-column="true"
show-row-number-column="true">
</lightning-datatable>
</div>
</lightning-card>
</template>
JavaScript:
import { LightningElement, wire, api } from 'lwc';
import { getRelatedListRecords } from 'lightning/uiRelatedListApi';
const COLUMNS = [
{ label: 'Name', fieldName: 'Name' },
{ label: 'Email', fieldName: 'Email' }
];
export default class RelatedListComponent extends LightningElement {
@api recordId;
recordCount;
records;
columns = COLUMNS;
@wire( getRelatedListRecords, {
parentRecordId: '$recordId',
relatedListId: 'Contacts',
fields: [ 'Contact.Id', 'Contact.Name', 'Contact.Email' ]
} )listInfo( { error, data } ) {
if ( data ) {
console.log( 'Data is', JSON.stringify( data ) );
let tempRecords = [];
data.records.forEach( obj => {
console.log( 'obj is', JSON.stringify( obj ) );
let tempRecord = {};
tempRecord.Id = obj.fields.Id.value;
tempRecord.Name = obj.fields.Name.value;
tempRecord.Email = obj.fields.Email.value;
tempRecords.push( tempRecord );
} );
this.records = tempRecords;
this.recordCount = data.count;
console.log( 'Records are ' + JSON.stringify( this.records ) );
} else if (error) {
this.records = undefined;
}
}
}
js-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>52.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__RecordPage</target>
</targets>
<targetConfigs>
<targetConfig targets="lightning__RecordPage">
<objects>
<object>Account</object>
</objects>
</targetConfig>
</targetConfigs>
</LightningComponentBundle>
Note:
I have configured the Lightning Web Component page in Account Lightning Record page.
Output:
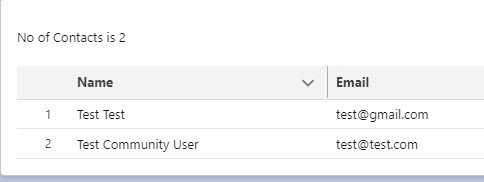