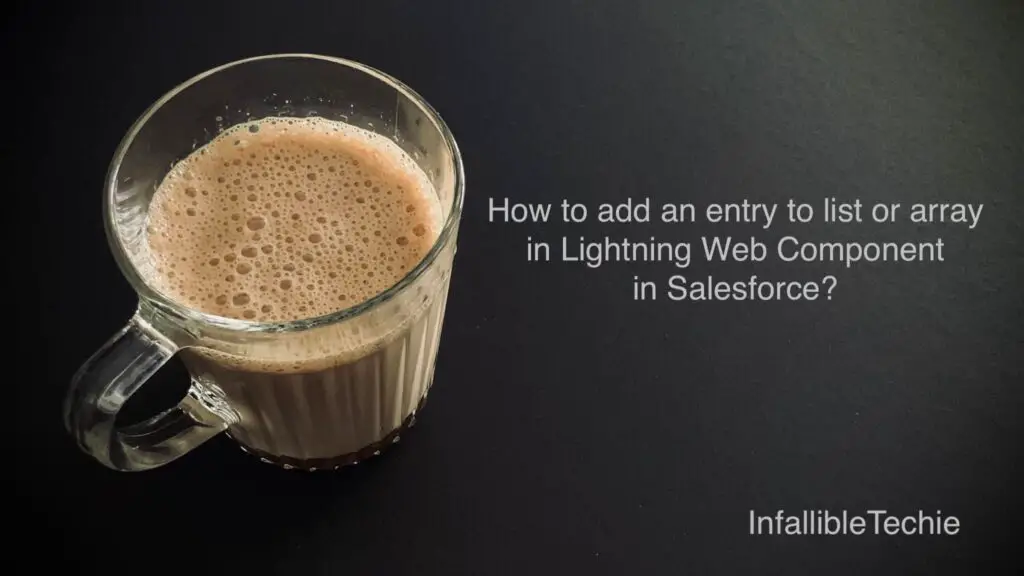
Avoid using push() method and construct new array so that the variables will be reactive to add an entry to a list or an array in Lightning Web Component in Salesforce.
HTML:
<template>
<lightning-card title="Array Example" icon-name="custom:custom14">
<lightning-datatable
key-field="Id"
data={listRecords}
columns={columns}
hide-checkbox-column="true">
</lightning-datatable>
<div class="slds-m-around_medium">
<lightning-button
variant="brand"
label="Add To Array"
title="Add To Array"
onclick={handleAddToArray}
class="slds-m-left_x-small">
</lightning-button>
</div>
</lightning-card>
</template>
JavaScript:
import { LightningElement } from 'lwc';
export default class ArrayExample extends LightningElement {
listRecords;
i = 0;
columns = [
{ label: 'Id', fieldName: 'Id' },
{ label: 'Test 1', fieldName: 'Test1' },
{ label: 'Test 2', fieldName: 'Test2' }
];
handleAddToArray() {
let newEntry = {
'Id' : this.i,
'Test1' : 'Test1 Val ' + this.i,
'Test2' : 'Test2 Val ' + this.i
};
if ( this.listRecords ) {
this.listRecords = [ ...this.listRecords, newEntry ];
} else {
this.listRecords = [ newEntry ];
}
this.i++;
}
}
js-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>52.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__Tab</target>
</targets>
</LightningComponentBundle>
Output: