HTML:
<template>
<lightning-card title="EmpApi Example" icon-name="custom:custom14">
<div class="slds-m-around_medium">
<lightning-button variant="success" label="Subscribe" title="Subscribe"
onclick={handleSubscribe} disabled={isSubscribeDisabled}
class="slds-m-left_x-small"></lightning-button>
<lightning-button variant="destructive" label="Unsubscribe" title="Unsubscribe"
onclick={handleUnsubscribe} disabled={isUnsubscribeDisabled}
class="slds-m-left_x-small"></lightning-button>
</div>
<lightning-datatable
key-field="ReplayId"
data={listEvents}
columns={columns}
hide-checkbox-column="true">
</lightning-datatable>
</lightning-card>
</template>
JavaScript:
import { LightningElement } from 'lwc';
import { subscribe, unsubscribe, onError } from 'lightning/empApi';
export default class SamplePlatformEventsListener extends LightningElement {
channelName = '/event/Test__e';
isSubscribeDisabled = false;
isUnsubscribeDisabled = !this.isSubscribeDisabled;
subscription = {};
listEvents;
columns = [
{ label: 'Serial No', fieldName: 'SerialNo' },
{ label: 'Replay Id', fieldName: 'ReplayId' },
{ label: 'Schema', fieldName: 'Schema' }
];
connectedCallback() {
this.registerErrorListener();
}
handleSubscribe() {
const messageCallback = ( response ) => {
console.log( 'New message received: ', JSON.stringify( response ) );
let val = {
'SerialNo' : response.data.payload.Serial_No__c,
'Schema' : response.data.schema,
'ReplayId' : response.data.event.replayId
};
console.log( 'Val is ' + JSON.stringify( val ) );
console.log( 'Events before assignment are ' + JSON.stringify( this.listEvents ) );
if ( this.listEvents ) {
this.listEvents = [ ...this.listEvents, val ];
} else {
this.listEvents = [ val ];
}
console.log( 'Events after assignment are ' + JSON.stringify( this.listEvents ) );
};
subscribe( this.channelName, -1, messageCallback ).then( response => {
console.log( 'Subscription request sent to: ', JSON.stringify( response.channel ) );
this.subscription = response;
this.toggleSubscribeButton( true );
});
}
handleUnsubscribe() {
this.toggleSubscribeButton( false );
unsubscribe( this.subscription, response => {
console.log( 'unsubscribe() response: ', JSON.stringify( response ) );
});
}
toggleSubscribeButton( enableSubscribe ) {
this.isSubscribeDisabled = enableSubscribe;
this.isUnsubscribeDisabled = !enableSubscribe;
}
registerErrorListener() {
onError( error => {
console.log( 'Received error from server: ', JSON.stringify( error ) );
});
}
}
js-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>52.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__Tab</target>
</targets>
</LightningComponentBundle>
Output:
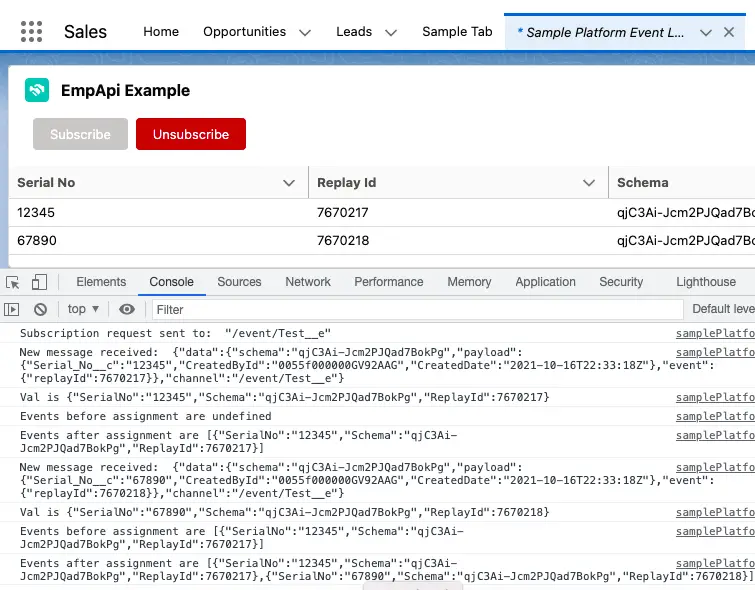