lightning-record-form can be used in the Salesforce Lightning Web Component for Picklist field inline editing.
Sample Code:
Apex Class:
public with sharing class AccountController {
@AuraEnabled( cacheable = true )
public static List< Account > fetchAccounts() {
return [ SELECT Id, Name, Industry, ParentId, RecordTypeId FROM Account LIMIT 10 ];
}
}
Lightning Web Component:
HTML:
<template>
<lightning-card title="Accounts">
<template iterator:it={records}>
<div key={it.value.Id}>
<lightning-record-form
record-id={it.value.Id}
object-api-name="Account"
fields={fields}
record-type-id={it.value.RecordTypeId}
columns="3"
mode="view">
</lightning-record-form>
</div>
</template>
</lightning-card>
</template>
JavaScript:
import { LightningElement, wire } from 'lwc';
import fetchAccounts from '@salesforce/apex/AccountController.fetchAccounts';
import NAME_FIELD from '@salesforce/schema/Account.Name';
import INDUSTRY_FIELD from '@salesforce/schema/Account.Industry';
import PARENT_FIELD from '@salesforce/schema/Account.ParentId';
export default class SampleLWC extends LightningElement {
records;
wiredRecords;
error;
fields = [ NAME_FIELD, INDUSTRY_FIELD, PARENT_FIELD ];
@wire( fetchAccounts )
wiredAccount( value ) {
this.wiredRecords = value;
const { data, error } = value;
if ( data ) {
this.records = data;
this.error = undefined;
} else if ( error ) {
this.error = error;
this.records = undefined;
}
}
}
js-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>50.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__Tab</target>
</targets>
</LightningComponentBundle>
Output:
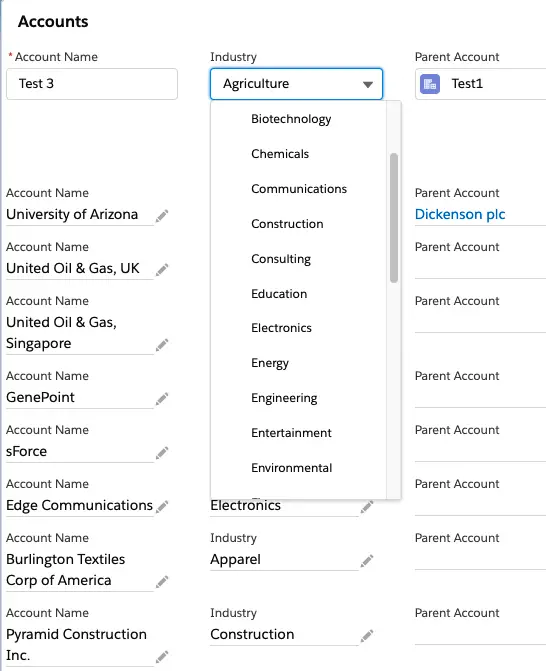