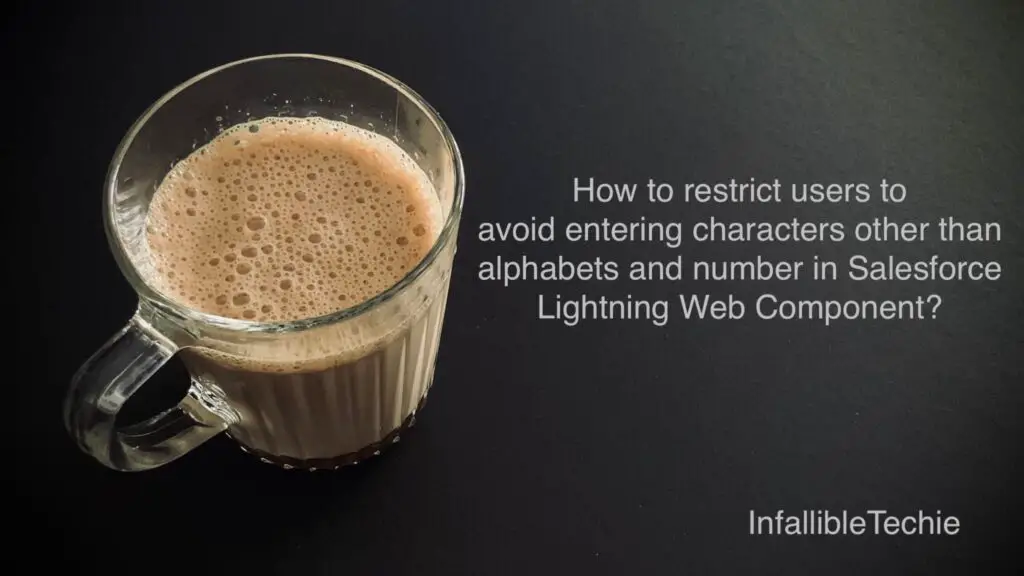
For Salesforce Lightning Web Component lighning-input Validation, pattern and message-when-pattern-mismatch attributes can be used.
pattern attribute in the lightning-input tag can be used to restrict users to avoid entering characters other than alphabets and number in Salesforce Lightning Web Component. Regular Expression should be used. In the following example, I have allowed alphabets and numbers alone.
Sample Code:
Lightning Web Component:
HTML:
<template>
<div class="slds-box slds-theme--default">
<lightning-input
type="text"
label="String Only"
pattern="[a-zA-z0-9]+"
message-when-pattern-mismatch="Special Characters not allowed"
onblur={handleBlur}>
</lightning-input>
<br/><br/>
<lightning-button
label="Store"
disabled={disableBool}>
</lightning-button>
</div>
</template>
JavaScript:
import { LightningElement } from 'lwc';
export default class sampleLightningWebComponent extends LightningElement {
disableBool = true;
handleBlur( event ) {
console.log(
event.target.value
);
let inputCmp = this.template.querySelector(
'lightning-input'
);
if ( inputCmp.checkValidity() ) {
this.disableBool = false;
} else {
this.disableBool = true;
}
}
}
js-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>59.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__Tab</target>
</targets>
</LightningComponentBundle>
Output:
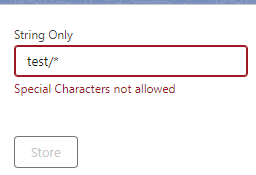