In the below example, if the account type is Customer – Direct and if the user tries to update any fields other than Industry and Description, it will throw an error.
Field Set:
Sample Code:
trigger AccountTrigger on Account ( before update ) {
Map < String, Schema.SObjectField > accountFieldsMap = new Map < String, Schema.SObjectField >();
Set < String > setFieldsToIgnore = new Set < String >();
for ( Schema.FieldSetMember f : SObjectType.Account.FieldSets.Fields_To_Excluded_for_Update.getFields() )
setFieldsToIgnore.add( f.getFieldPath() );
for ( Schema.SObjectField objField : Schema.SObjectType.Account.fields.getMap().values() ) {
Schema.DescribeFieldResult F = objField.getDescribe();
String strFieldName = F.getName();
if ( F.isUpdateable() && !setFieldsToIgnore.contains( strFieldName ) )
accountFieldsMap.put( strFieldName, objField );
}
for ( Account objAcc : trigger.new ) {
Account oldAcc = trigger.oldMap.get( objAcc.Id );
if ( objAcc.Type == 'Customer - Direct' && oldAcc.Type == 'Customer - Direct' ) {
List < String > fieldNames = new List < String >();
for ( String objField : accountFieldsMap.keySet() ) {
if ( objAcc.get( objField ) != oldAcc.get( objField ) )
fieldNames.add( accountFieldsMap.get( objField ).getDescribe().getLabel() );
}
if ( fieldNames.size() > 0 )
objAcc.addError( 'You cannot edit ' + String.join( fieldNames, ', ' ) );
}
}
}
Output:
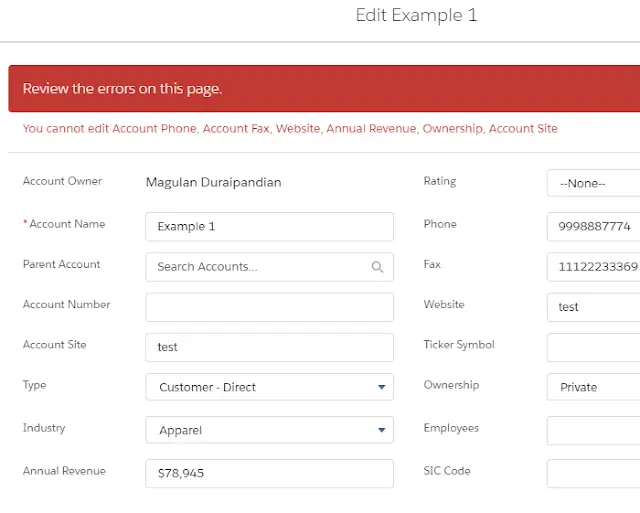