1. Add the Salesforce My Domain URL in your Remote Site Settings in Setup in the Source org.
Remote Site Setting:
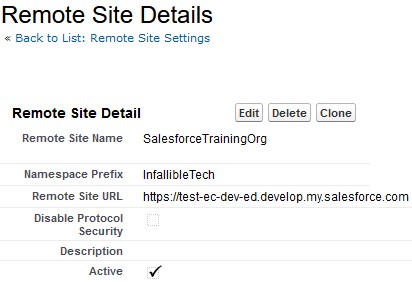
2. Create a Connected app in the target org. Make a note of the Consumer Key and Secret.
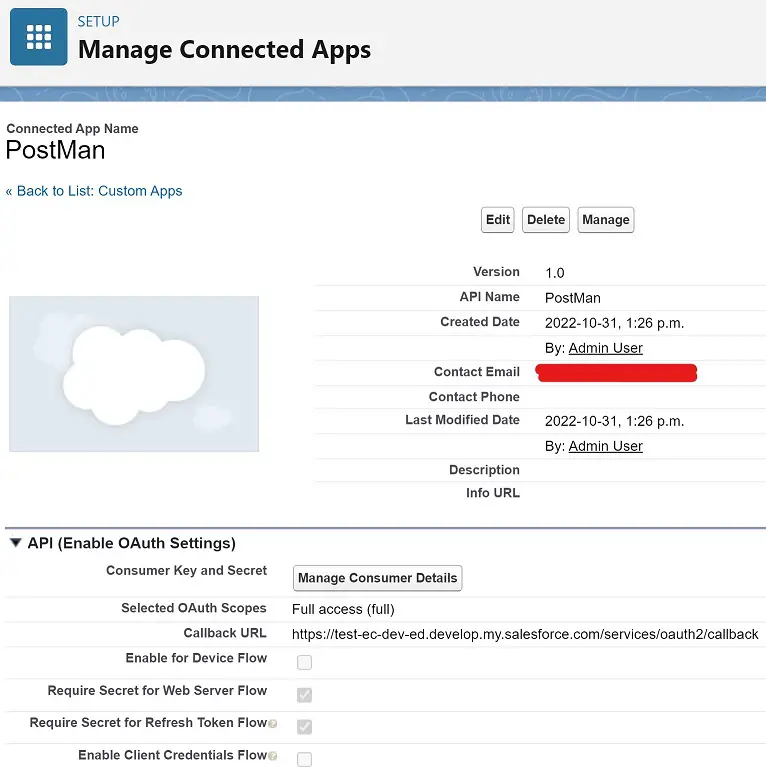
Sample Code:
HttpResponse response = new HttpResponse();
HttpRequest req = new HttpRequest();
Http objHttp = new Http();
String strEndPoint = '{YOUR_DOMAIN_URL}';
String strUsername = '{YOUR_USERNAME}';
String strPassword = '{YOUR_PASSWORD}';
String strClientId = '{YOUR_CONNECTED_APP_CONSUMER_KEY}';
String strClientSecret = '{YOUR_CONNECTED_APP_CONSUMER_SECRET}';
String strBody = 'username=' + strUsername;
strBody += '&password=' + strPassword;
strBody += '&client_id=' + strClientId;
strBody += '&client_secret=' + strClientSecret;
strBody += '&grant_type=password';
req.setHeader( 'Content-Type', 'application/x-www-form-urlencoded' );
req.setBody( strBody );
req.setEndpoint(
strEndPoint + '/services/oauth2/token'
);
req.setMethod( 'POST');
response = objHttp.send( req );
System.debug(
'Access Token Response is ' + response
);
if ( response.getStatusCode() == 200 ) {
Map < String, Object > resultMap = ( Map< String, Object> )JSON.deserializeUntyped( response.getBody() );
String strAccessToken = ( String )resultMap.get( 'access_token' );
System.debug(
'Access Token is ' + strAccessToken
);
req = new HttpRequest();
req.setHeader(
'Content-Type', 'application/json'
);
req.setHeader(
'Authorization',
'Bearer ' + strAccessToken
);
req.setEndpoint(
strEndPoint +
'/services/data/v57.0/sobjects/Account/'
);
strBody = '{ "Name" : "Testing", "Description" : "Testing" }';
req.setBody( strBody );
req.setMethod( 'POST' );
response = objHttp.send( req );
System.debug(
'Create Account Response is ' + response
);
if ( response.getStatusCode() == 200 ) {
System.debug(
'Response Body is ' + response.getBody()
);
}
}
Output:

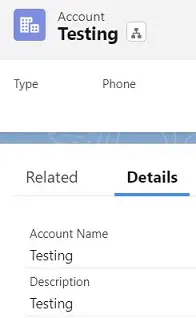