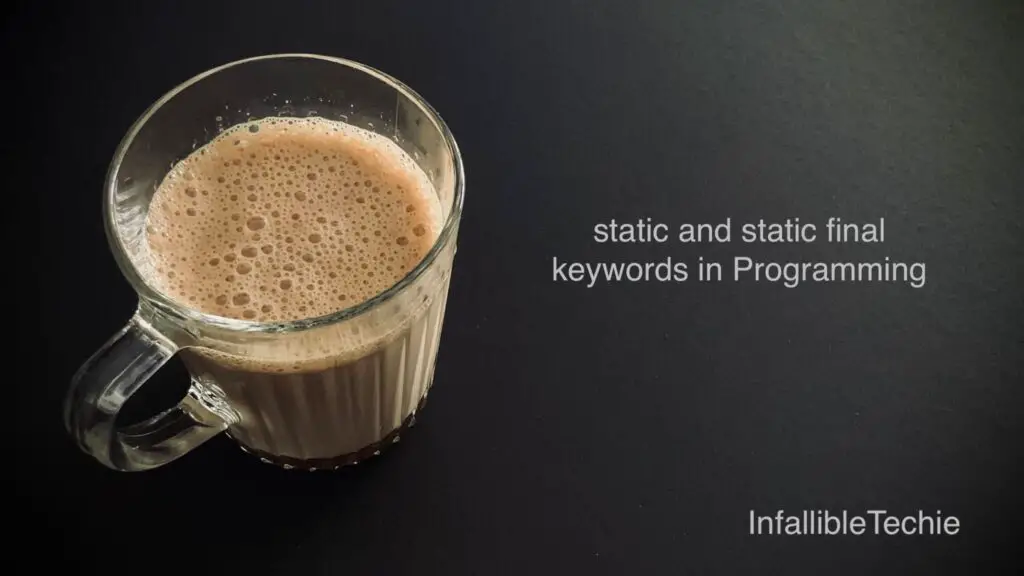
The static keyword is used to make the variable value the same for every instance of the class. We should use the class name to access the static variable. We cannot access the variable by initialising the class.
The final keyword used to make the variable unchangeable when the variable is assigned a value. So, it can never be changed once the value is assigned to it.
I have used Salesforce Apex for this example.
Sample Class:
public class SampleClass {
public static String strStaticVariable = 'Testing';
public static final String strFinalStaticVariable = 'Example';
}
Sample Code to test:
System.debug(
'Before Change: strStaticVariable is '
+ SampleClass.strStaticVariable
);
SampleClass.strStaticVariable = 'Value Changed';
System.debug(
'After Change: strStaticVariable is '
+ SampleClass.strStaticVariable
);
/*
The following exception will be thrown
since we are trying to assign value to a
already assigned static final variable.
Exception:
System.FinalException: Final variable has already been initialised
*/
SampleClass.strFinalStaticVariable = 'Test';