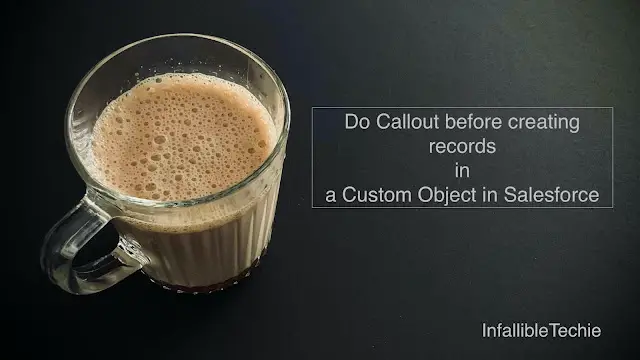
1. Sample Custom Object.
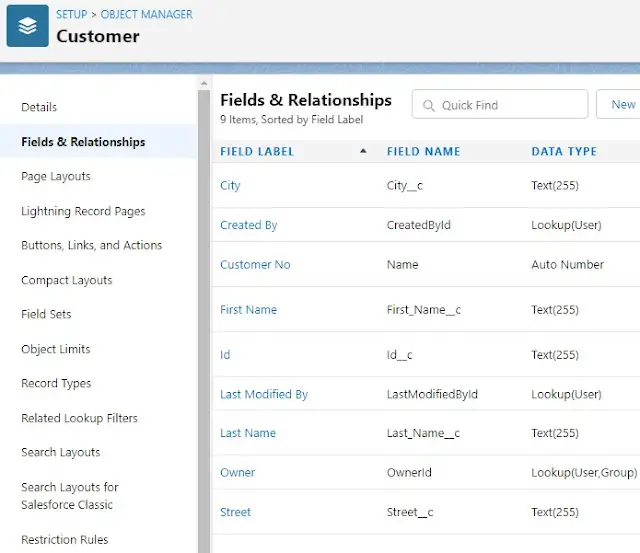
2. Create Remote Site Settings for API Endpoint.
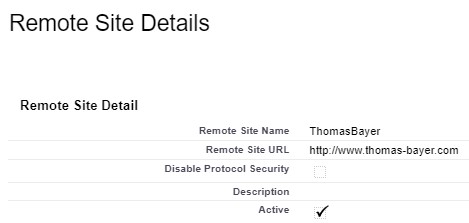
3. Sample Code to override the Standard New button in the custom object.
Apex Class:
public class CreateCustomerRecord {
@AuraEnabled( cacheable = true )
public static String fetchCustomerDetails( String strCustomerNo ) {
String endpoint = 'http://www.thomas-bayer.com/sqlrest/CUSTOMER/' + strCustomerNo + '/';
String strOutput = '{';
HTTP h = new HTTP();
HTTPRequest req = new HTTPRequest();
req.setEndPoint( endpoint );
req.setMethod( 'GET');
HTTPResponse res = h.send(req);
Dom.Document doc = res.getBodyDocument();
Dom.XMLNode customer = doc.getRootElement();
for ( Dom.XMLNode child : customer.getChildElements() ) {
strOutput += '"' + child.getName() + '" : "' + child.getText() + '", ';
}
strOutput = strOutput.removeEnd( ', ' );
strOutput += '}';
System.debug( 'strOutput ' + strOutput );
return strOutput;
}
}
Lightning Web Component:
HTML:
<template>
<lightning-card title="New Customer Record Creation">
<div class="slds-m-around_medium">
<lightning-input type="text" label="Enter Customer Number" onchange={onCustomerNoChange}></lightning-input>
<lightning-button label="Get Customer Details" onclick={fetchCustomerDetails}></lightning-button>
<lightning-record-edit-form
object-api-name="Customer__c"
onsuccess={handleSuccess}>
<lightning-messages></lightning-messages>
<lightning-input-field
field-name="First_Name__c"
disabled=true
value={strFirstName}>
</lightning-input-field>
<lightning-input-field
field-name="Last_Name__c"
disabled=true
value={strLastName}>
</lightning-input-field>
<lightning-input-field
field-name="Id__c"
disabled=true
value={strId}>
</lightning-input-field>
<lightning-input-field
field-name="Street__c"
disabled=true
value={strStreet}>
</lightning-input-field>
<lightning-input-field
field-name="City__c"
disabled=true
value={strCity}>
</lightning-input-field>
<lightning-button
type="submit"
name="submit"
label="Create Customer Record">
</lightning-button>
</lightning-record-edit-form>
</div>
</lightning-card>
</template>
JavaScript:
import { LightningElement, api } from 'lwc';
import fetchCustomerDetails from '@salesforce/apex/CreateCustomerRecord.fetchCustomerDetails';
import { ShowToastEvent } from 'lightning/platformShowToastEvent';
import { NavigationMixin } from 'lightning/navigation';
export default class CreateCustomerRecord extends NavigationMixin( LightningElement ) {
strCustomerNumber;
strId;
strFirstName;
strLastName;
strStreet;
strCity;
onCustomerNoChange( event ) {
this.strCustomerNumber = event.detail.value;
}
fetchCustomerDetails() {
console.log( 'strCustomerNumber is', this.strCustomerNumber );
fetchCustomerDetails( { strCustomerNo : this.strCustomerNumber } )
.then( result => {
console.log( 'Retrieved data is ' + result );
let customerRecord = JSON.parse( result );
this.strId = customerRecord.ID;
this.strFirstName = customerRecord.FIRSTNAME;
this.strLastName = customerRecord.LASTNAME;
this.strStreet = customerRecord.STREET;
this.strCity = customerRecord.CITY;
} )
.catch( error => {
console.error( 'Error Occured is', JSON.stringify( error ) );
} );
}
handleSuccess( event ) {
let recId = event.detail.id;
console.log( 'recId is', recId );
this.dispatchEvent(
new ShowToastEvent( {
title: 'Success',
message: event.detail.apiName + ' created.',
variant: 'success',
} )
);
this[ NavigationMixin.Navigate ]({
type: 'standard__recordPage',
attributes: {
recordId: recId,
objectApiName: 'Customer__c',
actionName: 'view'
}
});
}
}
js-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>54.0</apiVersion>
<isExposed>false</isExposed>
</LightningComponentBundle>
Lightning Aura Component:
<aura:component
implements="lightning:actionOverride,force:hasRecordId,force:hasSObjectName">
<c:createCustomerRecord/>
</aura:component>
4. Override the New button with the Lightning Aura Component.
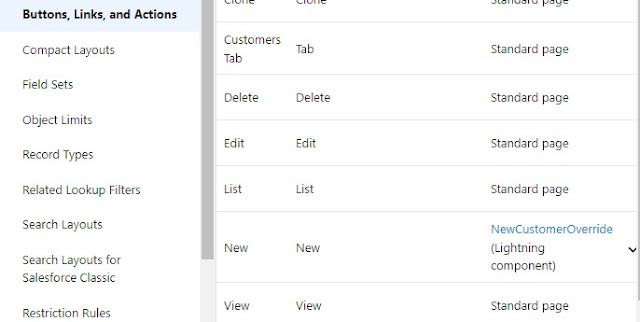
Output:
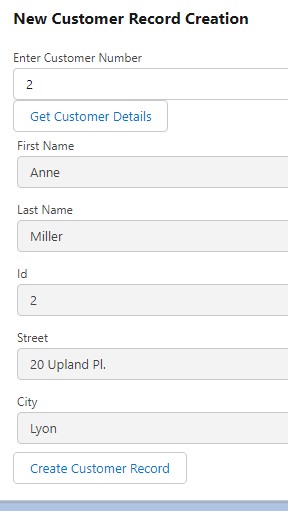
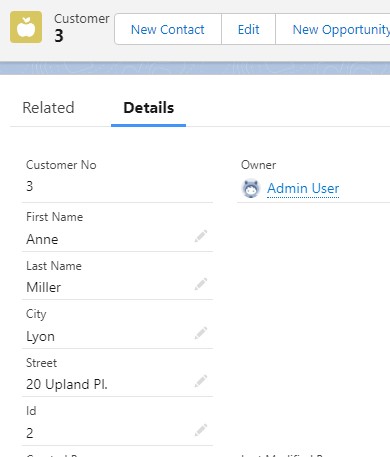
Video Reference: