1. Complete steps 1 through 10 from the following.
2
Use the same Apex Class from the above link.
Solution:
1. googleCaptcha is a re-usable LWC.This will fire events when the users click I’m not a robotand when the Captcha expires.
2. leadCreationForm is the parent LWC. This will handle the events from the googleCaptcha child component. When the Captcha verification is successful, the Create Lead Button will be enabled. If the Captcha verification fails or expires, the Create Lead Button will be disabled.
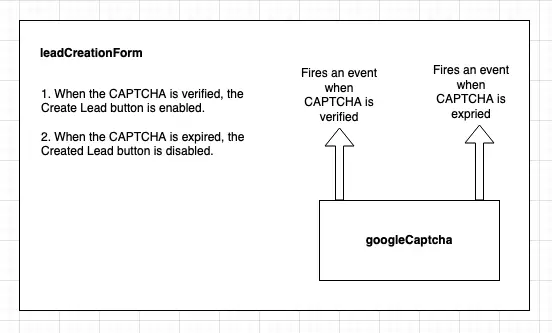
Sample Code:
googleCaptcha LWC:
HTML:
<template>
<div class="recaptchaCheckbox"></div>
</template>
JavaScript:
import { LightningElement } from 'lwc';
export default class GoogleCaptcha extends LightningElement {
connectedCallback() {
console.log( 'Inside Google Captcha Connected Callback' );
document.addEventListener( "grecaptchaVerified", ( e ) => {
console.log( 'Captcha Response from Verification is ' + e.detail.response );
let detailPayload = { value : false, response : e.detail.response };
this.dispatchEvent( new CustomEvent( 'captcha', { detail : detailPayload } ) );
});
document.addEventListener( "grecaptchaExpired", () => {
console.log( 'Listener Expired' );
this.dispatchEvent( new CustomEvent( 'captcha', { detail : { value : true } } ) );
} );
}
renderedCallback() {
console.log( 'Inside Google Captcha Rendered Callback' );
let divElement = this.template.querySelector( 'div.recaptchaCheckbox' );
console.log( 'Div Element is ' + JSON.stringify( divElement ) );
let payload = { element: divElement };
document.dispatchEvent(new CustomEvent( "grecaptchaRender", { "detail": payload } ) );
}
}
js-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>52.0</apiVersion>
<isExposed>true</isExposed>
</LightningComponentBundle>
leadCreationForm LWC:
HTML:
<template>
<lightning-card title="Lead Creation">
<lightning-record-edit-form object-api-name="Lead" onsubmit={handleSubmit}>
<lightning-messages></lightning-messages>
<lightning-input-field field-name="FirstName"></lightning-input-field>
<lightning-input-field field-name="LastName"></lightning-input-field>
<lightning-input-field field-name="Email"></lightning-input-field>
<lightning-input-field field-name="Phone"></lightning-input-field>
<lightning-input-field field-name="Company"></lightning-input-field>
<c-google-captcha oncaptcha={handleUpdate}></c-google-captcha><br/>
<lightning-button type="submit" label="Create Lead" variant="brand" disabled={verifiedBool}></lightning-button>
</lightning-record-edit-form>
</lightning-card>
</template>
JavaScript:
import { LightningElement } from 'lwc';
import insertRecord from '@salesforce/apex/RecaptchaController.insertRecord';
import {ShowToastEvent} from 'lightning/platformShowToastEvent';
export default class LeadCreationForm extends LightningElement {
verifiedBool = true;
captchaResponse
handleUpdate( event ) {
console.log( 'Updated value is ' + JSON.stringify( event.detail ) );
this.verifiedBool = event.detail.value;
if ( event.detail.response ) {
console.log( 'Response is ' + event.detail.response );
this.captchaResponse = event.detail.response;
}
}
handleSubmit( event ) {
let fields = event.detail.fields;
fields = Object.assign( { 'sobjectType': 'Lead' }, fields );
console.log( 'Fields are ' + JSON.stringify( fields ) );
console.log( 'Captcha Response is ' + JSON.stringify( this.captchaResponse ) );
event.preventDefault();
insertRecord( { record : fields, recaptchaResponse : this.captchaResponse } )
.then( result => {
this.isLoaded = false;
window.console.log('result ===> '+result);
this.strMessage = result;
this.dispatchEvent(
new ShowToastEvent( {
title: 'Lead Submission Result',
message: 'Lead ' + ( result.includes( 'Success' ) ? '' : 'not ' ) + 'Submitted Successfully',
variant: result.includes( 'Success' ) ? 'success' : 'error',
mode: 'sticky'
} )
);
})
.catch( error => {
this.dispatchEvent(
new ShowToastEvent( {
title: 'Error',
message: JSON.stringify( error ),
variant: 'error',
mode: 'sticky'
} )
);
} )
}
}
js-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>52.0</apiVersion>
<isExposed>true</isExposed>
<masterLabel>Lead Creation Form</masterLabel>
<targets>
<target>lightningCommunity__Page</target>
<target>lightningCommunity__Default</target>
</targets>
</LightningComponentBundle>
Output:
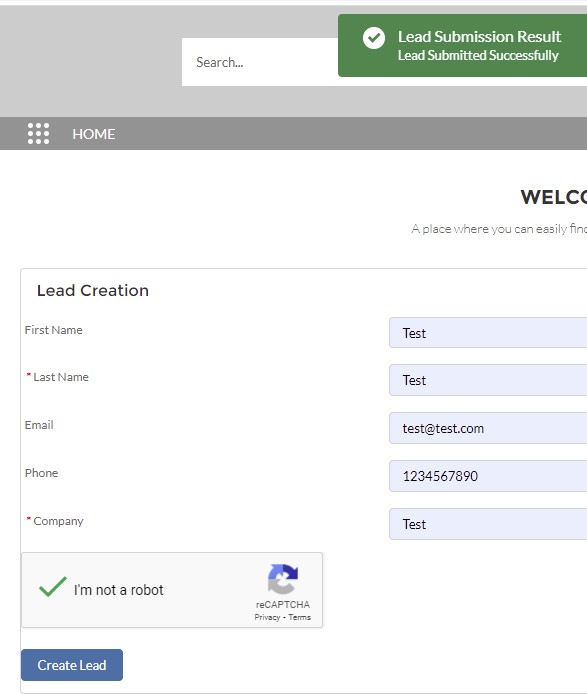