Use type: ‘boolean’ if you’re passing a boolean value to a column to display a checkmark for a true value.
Sample Code:
Apex Class:
public class AccountController {
@AuraEnabled( cacheable = true )
public static List< Account > fetchAccounts() {
return [ SELECT Id, Name, Industry, Is_Active__c FROM Account LIMIT 10 ];
}
}
Lightning Web Component:
HTML:
<template>
<lightning-card title="Accounts" icon-name="custom:custom63">
<div class="slds-m-around_medium">
<template if:true={accounts}>
<lightning-datatable
key-field="Id"
data={accounts}
columns={columns}
hide-checkbox-column="true"
show-row-number-column="true"
onrowaction={handleRowAction}>
</lightning-datatable>
</template>
<template if:true={error}>
{error}>
</template>
</div>
</lightning-card>
</template>
JavaScript:
import { LightningElement, wire } from 'lwc';
import fetchAccounts from '@salesforce/apex/AccountController.fetchAccounts';
import { NavigationMixin } from 'lightning/navigation';
const actions = [
{ label: 'View', name: 'view' },
{ label: 'Edit', name: 'edit' },
];
const columns = [
{ label: 'Name', fieldName: 'Name' },
{ label: 'Industry', fieldName: 'Industry' },
{ label: 'Is Active?', fieldName: 'Is_Active__c', type: 'boolean' },
{
type: 'action',
typeAttributes: { rowActions: actions },
},
];
export default class SampleLWC extends NavigationMixin( LightningElement ) {
accounts;
error;
columns = columns;
@wire( fetchAccounts )
wiredAccount( { error, data } ) {
if ( data ) {
this.accounts = data;
this.error = undefined;
} else if ( error ) {
this.error = error;
this.accounts = undefined;
}
}
handleRowAction( event ) {
const actionName = event.detail.action.name;
const row = event.detail.row;
switch ( actionName ) {
case 'view':
this[NavigationMixin.Navigate]({
type: 'standard__recordPage',
attributes: {
recordId: row.Id,
actionName: 'view'
}
});
break;
case 'edit':
this[NavigationMixin.Navigate]({
type: 'standard__recordPage',
attributes: {
recordId: row.Id,
objectApiName: 'Account',
actionName: 'edit'
}
});
break;
default:
}
}
}
JavaScript-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>50.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__Tab</target>
</targets>
</LightningComponentBundle>
Output:
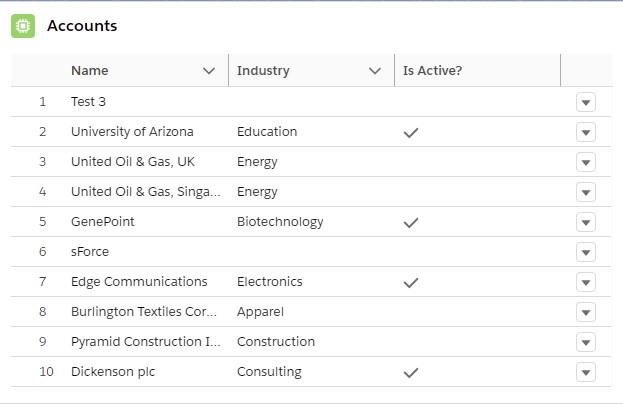