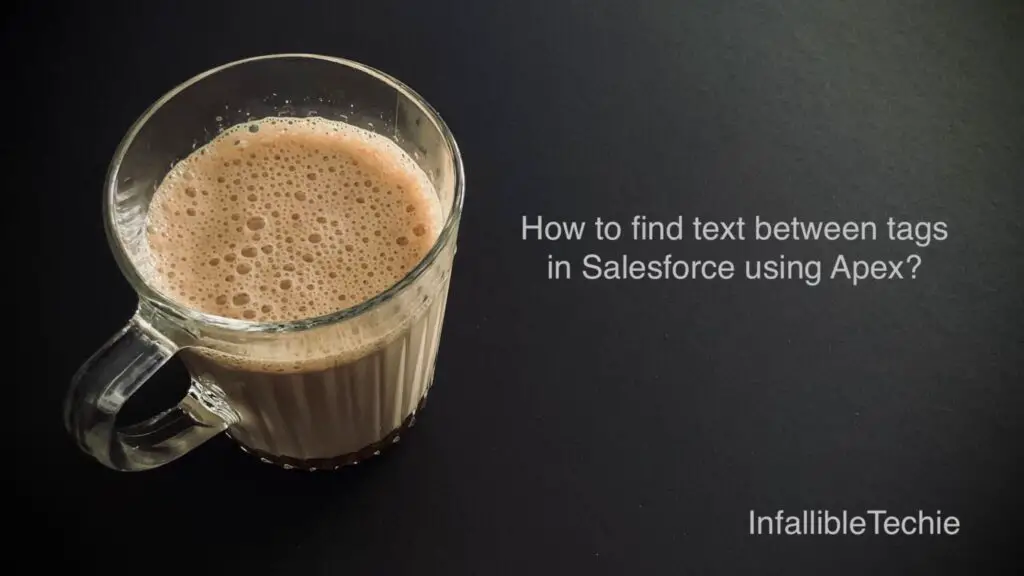
RegEx can be used to find text between tags in Salesforce using Apex.
Sample Code:
String str = '<test>abc</test><test>xyz</test>';
Pattern TAG_REGEX = Pattern.compile(
'<test>[a-z]*</test>'
);
Matcher matcher = TAG_REGEX.matcher(
str
);
while ( matcher.find() ) {
String strOutput = matcher.group().replace(
'<test>', ''
).replace(
'</test>', ''
);
System.debug(
strOutput
);
}
Output:
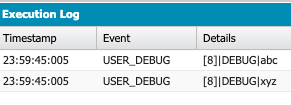
Sample Code with Capital Letters and Space:
String str = '<test>abc ABC+</test><test>xyz XYZ-</test>';
Pattern TAG_REGEX = Pattern.compile(
'<test>[a-zA-Z0-9\\s+-]*</test>'
);
Matcher matcher = TAG_REGEX.matcher(
str
);
while ( matcher.find() ) {
String strOutput = matcher.group().replace(
'<test>', ''
).replace(
'</test>', ''
);
System.debug(
strOutput
);
}
Output:
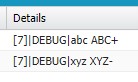