The Comparable interface adds sorting support for Lists that contain non-primitive types, that is, Lists of user-defined types.
To add List sorting support for your Apex class, you must implement the Comparable interface with its compareTo method in your class.
When a class implements the Comparable interface it has to implement a method called compareTo(). In that method the code has to be written to decide if two objects match or if one is larger than the other.
Sample Code:
Visualforce page:
<apex:page showHeader="false" controller="Sample" >
<apex:form >
<apex:pageBlock >
<apex:pageblockTable value="{!StudList}" var="S">
<apex:column value="{!S.StudentName}"/>
<apex:column value="{!S.StudentAge}"/>
</apex:pageblockTable>
</apex:pageBlock>
</apex:form>
</apex:page>
Apex Controller:
global class Sample {
public List<Student> StudList {get;set;}
public Sample() {
StudList = new List<Student>();
Student s1 = new Student('Ganesh', 12);
StudList.add(s1);
Student s2 = new Student('Arun', 82);
StudList.add(s2);
Student s3 = new Student('Vignesh', 32);
StudList.add(s3);
Student s4 = new Student('Younis', 55);
StudList.add(s4);
Student s5 = new Student('Azhar', 42);
StudList.add(s3);
StudList.sort();
}
//wrapper class for Comparable Interface
global class Student implements Comparable {
global String StudentName {get;set;}
global Integer StudentAge {get; set;}
global Student(String Name, Integer Age) {
StudentName = Name;
StudentAge = Age;
}
global Integer compareTo(Object ObjToCompare) {
return StudentName.CompareTo(((Student)ObjToCompare).StudentName);
}
}
}
Output:
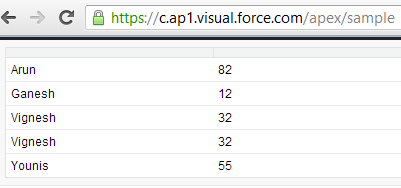