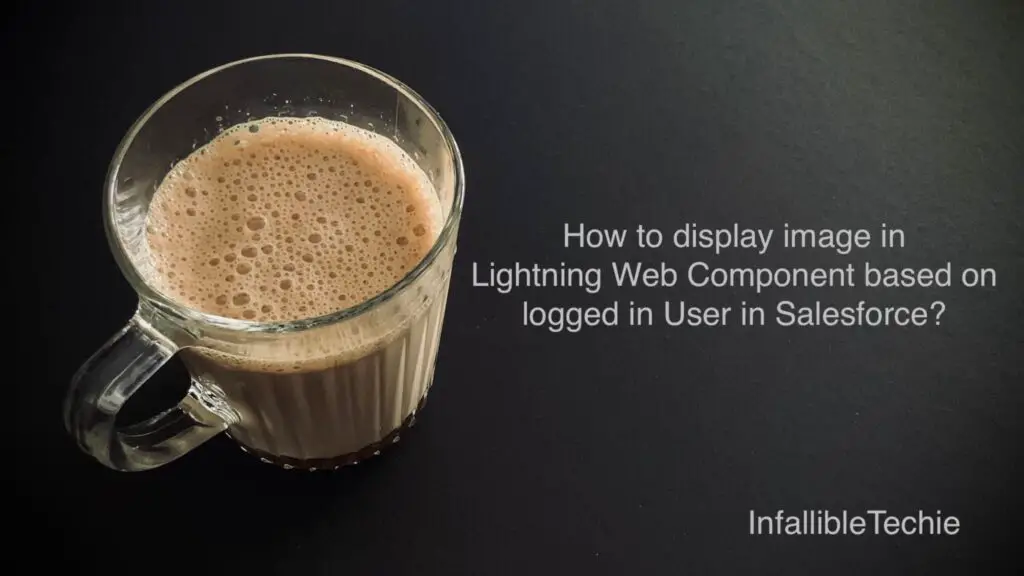
HTML img tag can be used to display image in Salesforce Lightning Web Component.
In the following example, I have used Static Resource to store the image. Image__c is a custom field in User object to store the Image URL for the user.
1. Use Static Resource to store Image if needed:
2. Custom URL Field in User object to store the Image URL:
3. Sample Code:
Lightning Web Component:
HTML:
<template>
<lightning-card>
<b>{name} - </b>
<img
src={imageURL}
alt="User Image"
height="100px"
width="100px"/>
</lightning-card>
</template>
JavaScript:
import { LightningElement, wire } from 'lwc';
import IdOfUser from '@salesforce/user/Id';
import { getRecord } from 'lightning/uiRecordApi';
import { ShowToastEvent } from 'lightning/platformShowToastEvent';
const FIELDS = [
'User.Name',
'User.Image__c'
];
export default class UserImage extends LightningElement {
userId = IdOfUser;
name;
imageURL;
connectedCallback() {
console.log( 'Id of the user is ' + this.userId );
console.log( 'Fields are ' + JSON.stringify( FIELDS ) );
}
@wire(getRecord, { recordId: '$userId', fields: FIELDS })
wiredRecord({ error, data }) {
if ( error ) {
let message = 'Unknown error';
if (Array.isArray(error.body)) {
message = error.body.map(e => e.message).join(', ');
} else if (typeof error.body.message === 'string') {
message = error.body.message;
}
this.dispatchEvent(
new ShowToastEvent({
title: 'Error loading Account',
message,
variant: 'error',
}),
);
} else if ( data ) {
console.log( 'Data is ' + JSON.stringify( data ) );
this.name = data.fields.Name.value;
this.imageURL = data.fields.Image__c.value;
}
}
}
JS-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>52.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__Tab</target>
</targets>
</LightningComponentBundle>
Output:
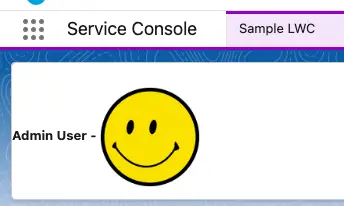