Sample Code:
Apex Class:
public with sharing class ExistingFilesController {
@AuraEnabled( cacheable = true )
public static List < ContentDocument > fetchContentDocuments( String strRecordId ) {
List < ContentDocument > listContentDocs = new List < ContentDocument >();
for ( ContentDocumentLink objCDL : [ SELECT Id, ContentDocument.Title, ContentDocumentId, LinkedEntityId FROM ContentDocumentLink WHERE LinkedEntityId =: strRecordId ] ) {
listContentDocs.add( new ContentDocument( Id = objCDL.ContentDocumentId, Title = objCDL.ContentDocument.Title ) );
}
return listContentDocs;
}
}
Lightning Web Component:
HTML:
<template>
<template if:true={recordsPresent}>
<lightning-card>
<lightning-datatable
key-field="Id"
data={listContentDocs}
columns={columns}
hide-checkbox-column="true"
show-row-number-column="true"
onrowaction={handleRowAction}>
</lightning-datatable>
</lightning-card>
</template>
</template>
JavaScript:
import { api, LightningElement, wire } from 'lwc';
import fetchContentDocuments from '@salesforce/apex/ExistingFilesController.fetchContentDocuments';
import { NavigationMixin } from 'lightning/navigation';
const actions = [
{ label: 'View', name: 'view' }
];
export default class ExistingFiles extends NavigationMixin( LightningElement ) {
@api
recordId;
columns = [
{ label: 'Id', fieldName: 'Id' },
{ label: 'Title', fieldName: 'Title' },
{
type: 'action',
typeAttributes: { rowActions: actions },
}
];
recordsPresent = false;
@wire( fetchContentDocuments, { strRecordId: '$recordId' } )
wiredRecs( { error, data } ) {
if ( data ) {
console.log( 'Records are ' + JSON.stringify( data ) );
this.listContentDocs = data;
this.recordsPresent = true;
} else if ( error ) {
console.log( 'Error ' + JSON.stringify( error ) );
}
}
handleRowAction( event ) {
console.log( 'Inside Handle Row Action' );
const row = event.detail.row;
this[ NavigationMixin.Navigate ]( {
type: 'standard__recordPage',
attributes: {
recordId: row.Id,
actionName: 'view'
}
} );
}
}
JS-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>52.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightningCommunity__Page</target>
<target>lightningCommunity__Default</target>
</targets>
<targetConfigs>
<targetConfig targets="lightningCommunity__Default">
<property
name="recordId" type="String"
label="Record Id" />
</targetConfig>
</targetConfigs>
</LightningComponentBundle>
Experience Builder Configuration:
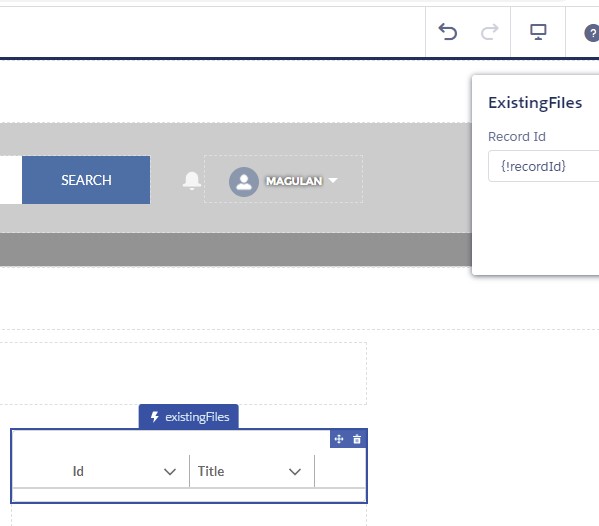
Output:
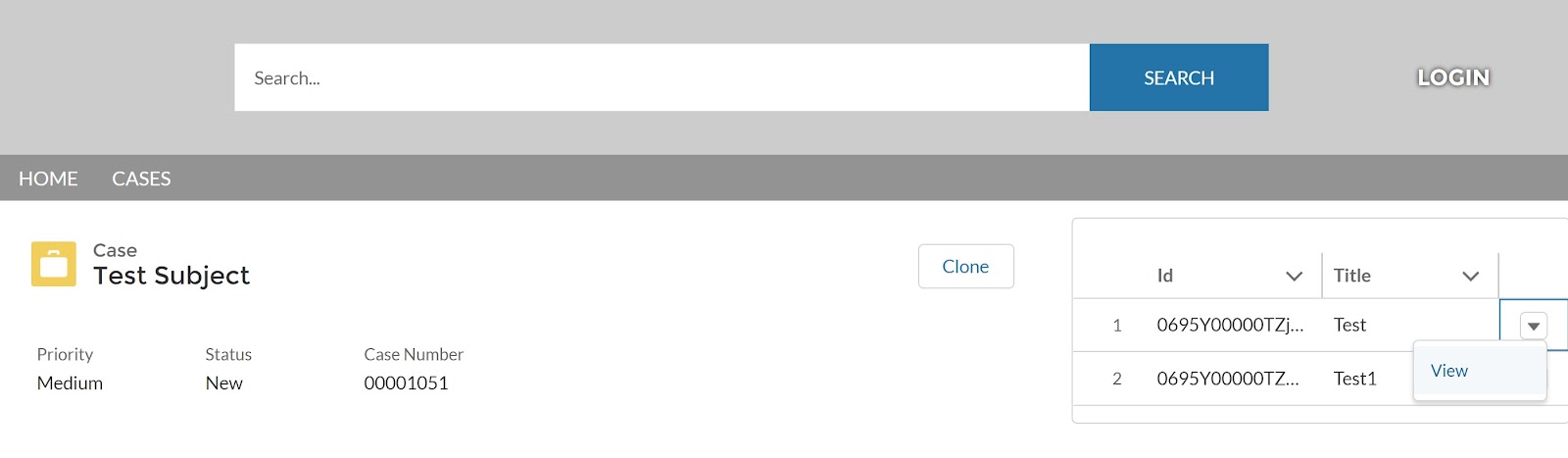
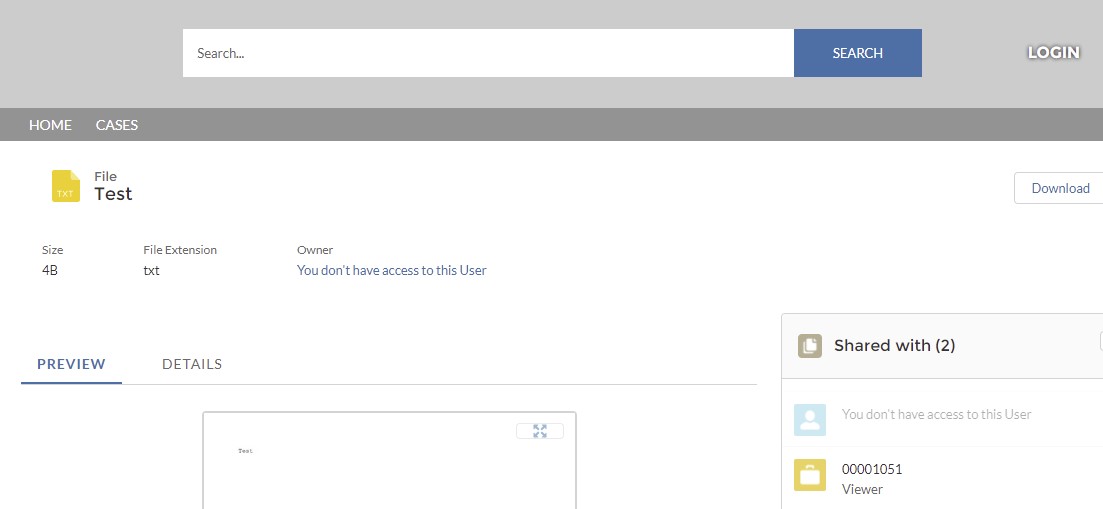