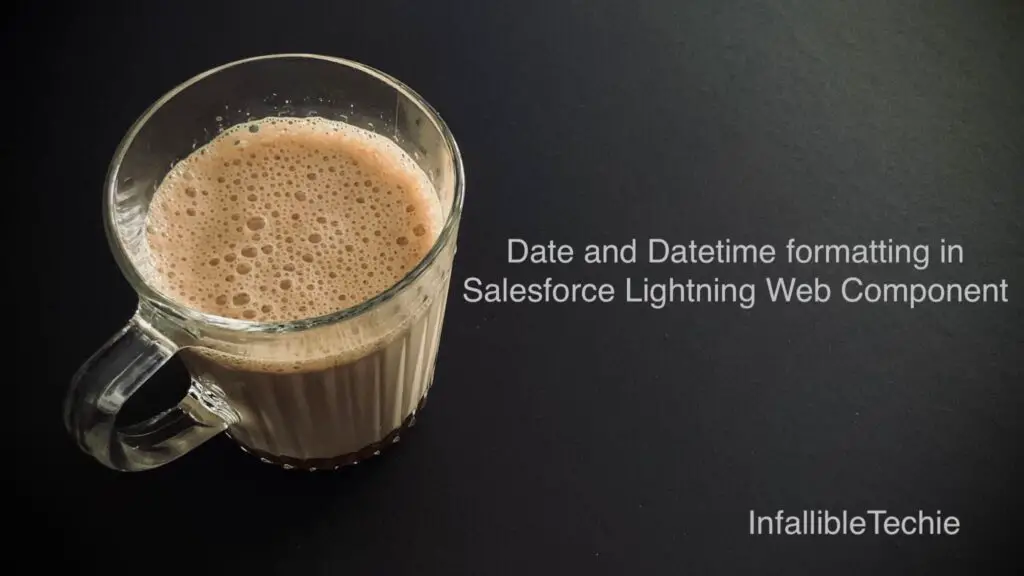
Sample Code:
HTML:
<template>
<div class="slds-box slds-theme--default">
<table class="slds-table slds-table_cell-buffer slds-table_bordered slds-table_striped">
<thead>
<tr class="slds-line-height_reset">
<th class="" scope="col">
Account Name
</th>
<th class="" scope="col">
Created Date
</th>
<th class="" scope="col">
Effective Date
</th>
</tr>
</thead>
<tbody>
<template iterator:it={records}>
<tr class="slds-hint-parent" key = {it.value.Id}>
<td data-label="Account Name">
<div class="slds-cell-wrap">{it.value.Name}</div>
</td>
<td data-label="Account Number">
{it.value.CreatedDate}
</td>
<td data-label="Owner">
<div class="slds-cell-wrap">{it.value.Effective_Date__c}</div>
</td>
</tr>
</template>
</tbody>
</table>
</div>
</template>
JavaScript:
import { LightningElement, wire } from 'lwc';
import fetchAccounts from '@salesforce/apex/AccountController.fetchAccounts';
export default class Sample extends LightningElement {
records;
error;
@wire( fetchAccounts )
wiredAccount( { error, data } ) {
if ( data ) {
let rows = JSON.parse( JSON.stringify( data ) );
console.log( 'Rows are ' + JSON.stringify( rows ) );
const options = {
year: 'numeric', month: 'numeric', day: 'numeric',
hour: 'numeric', minute: 'numeric', second: 'numeric',
hour12: false
};
for ( let i = 0; i < rows.length; i++ ) {
let dataParse = rows[ i ];
if ( dataParse.Effective_Date__c ) {
let dt = new Date( dataParse.Effective_Date__c );
dataParse.Effective_Date__c = new Intl.DateTimeFormat( 'en-US' ).format( dt );
}
if ( dataParse.CreatedDate ) {
let dt = new Date( dataParse.CreatedDate );
dataParse.CreatedDate = new Intl.DateTimeFormat( 'en-US', options ).format( dt );
}
}
this.records = rows;
this.error = undefined;
} else if ( error ) {
this.error = error;
this.records = undefined;
}
}
}
Apex Class:
public with sharing class AccountController {
@AuraEnabled( cacheable = true )
public static List< Account > fetchAccounts() {
return [ SELECT Id, Name, CreatedDate, Effective_Date__c FROM Account LIMIT 10 ];
}
}
JS-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>55.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__Tab</target>
</targets>
</LightningComponentBundle>
Output: