Sample Code:
Apex Class:
public class AccountListController {
@AuraEnabled
public static List < Account > fetchAccounts( Integer intOffSet ) {
List < Account > listAccounts = new List < Account >();
System.debug(
'intOffSet is ' + intOffSet
);
if ( intOffSet != null ) {
listAccounts = [
SELECT Id, Name, Industry, AccountNumber
FROM Account
LIMIT 5 OFFSET : Integer.valueOf( intOffSet )
];
}
System.debug(
'listAccounts is ' +
listAccounts
);
return listAccounts;
}
}
Lightning Web Component:
HTML:
<template>
<div style="height:200px;">
<lightning-card title="Accounts" icon-name="custom:custom63">
<template if:true={accountRecords}>
<div style="height:300px;">
<lightning-datatable
key-field="Id"
data={accountRecords}
columns={columns}
show-row-number-column
enable-infinite-loading={infiniteLoadingBool}
is-loading={isLoadingBool}
onloadmore={onLoadMore}
onrowselection={onRowSelection}>
</lightning-datatable>
</div>
</template>
<template if:true={error}>
Error is {error}
</template>
</lightning-card>
</div>
</template>
JavaScript:
import { LightningElement } from 'lwc';
import fetchAccounts from '@salesforce/apex/AccountListController.fetchAccounts';
const COLUMNS = [
{ label: 'Name', fieldName: 'Name' },
{ label: 'Industry', fieldName: 'Industry' },
{ label: 'Account Number', fieldName: 'AccountNumber' }
];
export default class sampleLightningWebComponent extends LightningElement {
accountRecords = [];
error;
columns = COLUMNS;
recordSize = 0;
isLoadingBool = true;
infiniteLoadingBool = true;
connectedCallback() {
console.log( 'Inside connected callback' );
this.onLoadMore();
}
onLoadMore() {
console.log(
'recordSize is ',
this.recordSize
);
fetchAccounts( { intOffSet : this.recordSize } )
.then( result => {
console.log(
'result is ',
JSON.stringify( result )
);
if ( result.length > 0 ) {
if ( this.recordSize > 0 ) {
this.accountRecords = [ ...this.accountRecords, ...result ];
console.log(
'No of Account Records is ',
this.accountRecords.length
);
} else {
this.accountRecords = result;
}
console.log(
'accountRecords are ',
JSON.stringify( this.accountRecords )
);
} else {
this.infiniteLoadingBool = false;
}
this.isLoadingBool = false;
})
.catch( error => {
console.log(
'error is ',
JSON.stringify( error )
);
this.error = JSON.stringify( error );
});
this.recordSize = this.recordSize + 5;
}
onRowSelection( event ) {
const selectedRows = event.detail.selectedRows;
console.log(
'selectedRows are ',
JSON.stringify( selectedRows )
);
}
}
js-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>56.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__Tab</target>
</targets>
</LightningComponentBundle>
Output:
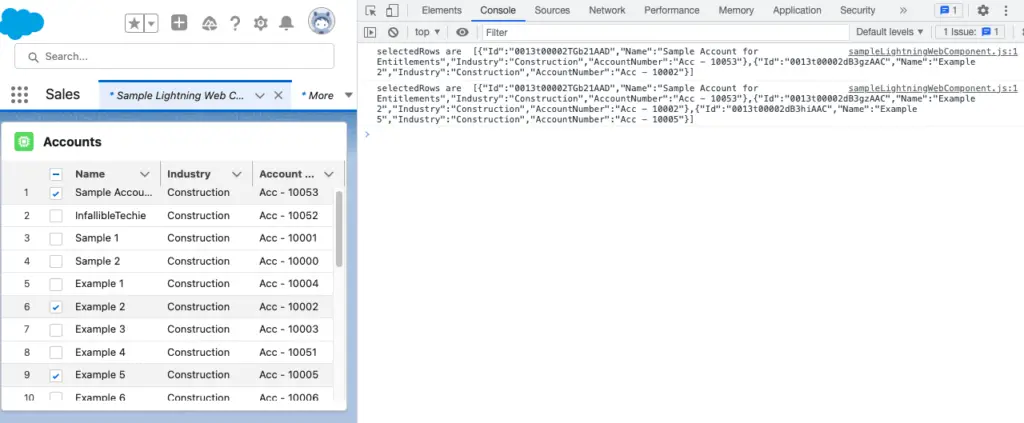