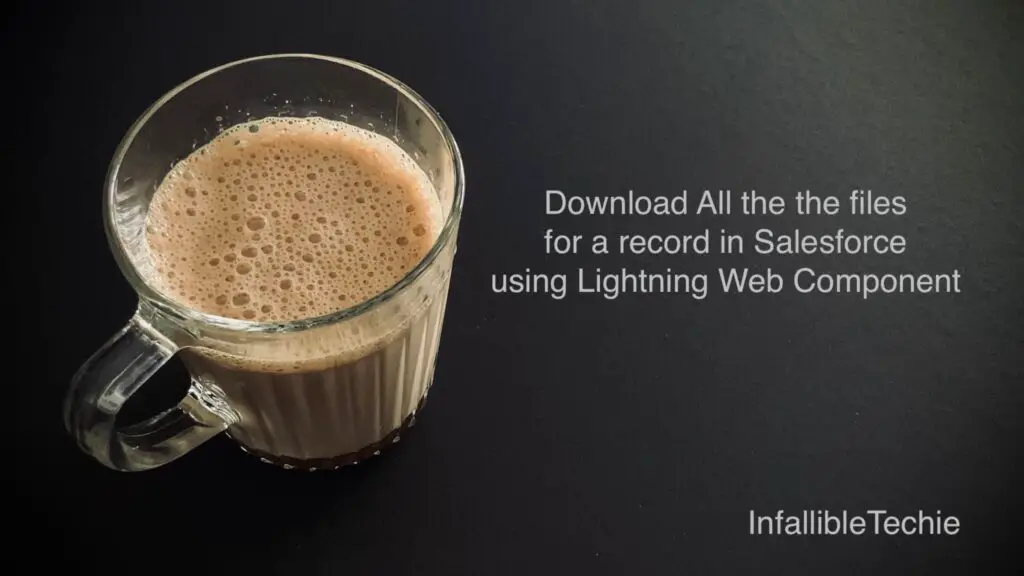
Using the following Lightning Web Component, we can create a Quick Action to Download All the the files for a record in Salesforce.
Sample code:
Apex Class:
public with sharing class MassDownloadFilesController {
@AuraEnabled( cacheable = true )
public static List < Id > fetchRelatedFiles( Id strRecordId ) {
List < Id > listFileIds = new List < Id >();
for ( ContentDocumentLink objCDL: [
SELECT ContentDocument.LatestPublishedVersionId
FROM ContentDocumentLink
WHERE LinkedEntityId =: strRecordId
] ) {
listFileIds.add( objCDL.ContentDocument.LatestPublishedVersionId );
}
return listFileIds;
}
}
Lightning Web Component:
HTML:
<template>
</template>
JavaScript:
import { LightningElement, api } from 'lwc';
import { ShowToastEvent } from 'lightning/platformShowToastEvent';
import fetchRelatedFiles from '@salesforce/apex/MassDownloadFilesController.fetchRelatedFiles';
import { NavigationMixin } from 'lightning/navigation';
export default class MassDownloadFiles extends NavigationMixin ( LightningElement ) {
@api recordId;
@api invoke() {
fetchRelatedFiles( { strRecordId : this.recordId } )
.then( result => {
console.log( 'result is', JSON.stringify( result ) );
if ( result.length > 0 ) {
let filesDownloadUrl = '/sfc/servlet.shepherd/version/download';
result.forEach( item => {
filesDownloadUrl += '/' + item
});
console.log( 'filesDownloadUrl is', filesDownloadUrl );
this[ NavigationMixin.Navigate ]( {
type: 'standard__webPage',
attributes: {
url: filesDownloadUrl
}
}, false );
this.dispatchEvent(
new ShowToastEvent( {
title: 'File(s) Download',
message: 'File(s) Download Successfully!!!',
variant: 'success'
} ),
);
} else {
this.dispatchEvent(
new ShowToastEvent({
title: 'File(s) Download',
message: 'File(s) Download failed!!!',
variant: 'error'
}),
);
}
} )
.catch( error => {
this.dispatchEvent(
new ShowToastEvent({
title: 'File(s) Download Failed',
message: 'System Error Occurred',
variant: 'error'
}),
);
} );
}
}
JS-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>55.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__RecordAction</target>
<target>lightning__RecordPage</target>
</targets>
<targetConfigs>
<targetConfig targets="lightning__RecordAction">
<actionType>Action</actionType>
</targetConfig>
</targetConfigs>
</LightningComponentBundle>
Quick Action:
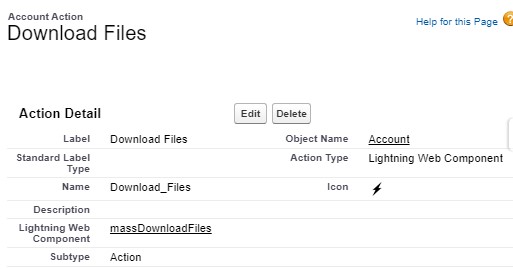
Output:
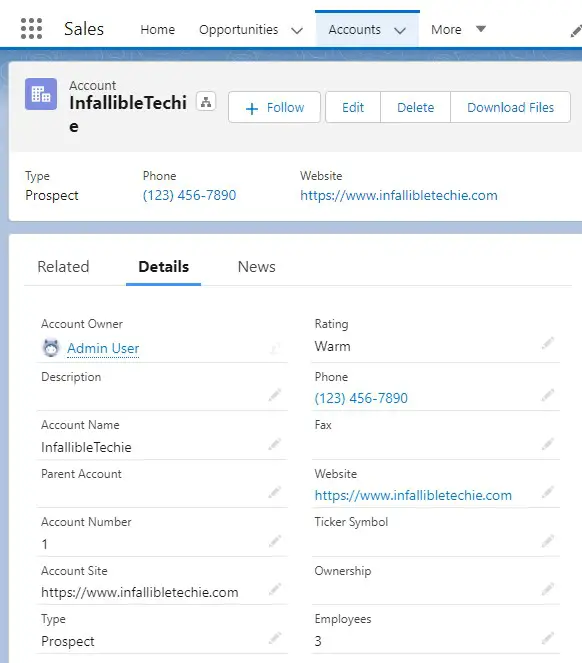