The @api decorator in the child component exposes a property, making it public, so that the parent component can update it.
Similarly, @api decorator function in child component can expose it as public to call it from the parent component.
Sample Code:
childLWC.html:
<template>
Value entered in Parent Component is {strOutput}
</template>
childLWC.js:
import { api, LightningElement } from 'lwc';
export default class ChildLWC extends LightningElement {
@api
strOutput;
}
childLWC.js-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>50.0</apiVersion>
<isExposed>false</isExposed>
</LightningComponentBundle>
parentLWC.html:
<template>
<div class="slds-box slds-theme_default">
Enter value: <lightning-input
type="text"
value={strInput}
onchange={handleChange}></lightning-input> <br>
<c-child-l-w-c str-output={strInput}></c-child-l-w-c>
</div>
</template>
parentLWC.js:
import { LightningElement } from 'lwc';
export default class ParentLWC extends LightningElement {
strInput;
handleChange( event ) {
this.strInput = event.target.value;
}
}
parentLWC.js-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>50.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__Tab</target>
</targets>
</LightningComponentBundle>
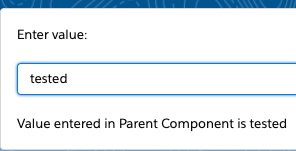