Use state attribute to pass query parameters or URL parameters in lightning navigation.
Sample Code:
Apex Class:
public with sharing class AccountController {
@AuraEnabled( cacheable = true )
public static List< Account > fetchAccounts() {
return [ SELECT Id, Name, Industry FROM Account LIMIT 10 ];
}
}
Publishing LWC Component:
HTML:
<template>
<lightning-card title = "Accounts" icon-name = "custom:custom63">
<div class = "slds-m-around_medium">
<template if:true = {accounts}>
<div style="height: 300px;">
<lightning-datatable key-field="Id"
data={accounts}
columns={columns}
hide-checkbox-column="true"
show-row-number-column="true"
onrowaction={handleRowAction}>
</lightning-datatable>
</div>
</template>
<template if:true = {error}>
{error}>
</template>
</div>
</lightning-card>
</template>
JavaScript:
import { LightningElement, track, wire } from 'lwc';
import fetchAccounts from '@salesforce/apex/AccountController.fetchAccounts';
import { NavigationMixin } from 'lightning/navigation';
const actions = [
{ label: 'View', name: 'view' },
{ label: 'Edit', name: 'edit' },
];
const columns = [
{ label: 'Name', fieldName: 'Name' },
{ label: 'Industry', fieldName: 'Industry' },
{
type: 'action',
typeAttributes: { rowActions: actions },
},
];
export default class LightningDataTableLWC extends NavigationMixin( LightningElement ) {
@track accounts;
@track error;
@track columns = columns;
@wire(fetchAccounts)
accountData( { error, data } ) {
if ( data ) {
this.accounts = data;
this.error = undefined;
} else if ( error ) {
if ( Array.isArray( error.body ) )
console.log( 'Error is ' + error.body.map( e => e.message ).join( ', ' ) );
else if ( typeof error.body.message === 'string' )
console.log( 'Error is ' + error.body.message );
}
}
handleRowAction( event ) {
const actionName = event.detail.action.name;
const row = event.detail.row;
switch ( actionName ) {
case 'view':
this[NavigationMixin.Navigate]({
type: 'standard__recordPage',
attributes: {
recordId: row.Id,
actionName: 'view'
},
state: {
c__sample: 'testing'
}
});
break;
case 'edit':
this[NavigationMixin.Navigate]({
type: 'standard__recordPage',
attributes: {
recordId: row.Id,
objectApiName: 'Account',
actionName: 'edit'
}
});
break;
default:
}
}
}
meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>49.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__Tab</target>
</targets>
</LightningComponentBundle>
Subscribing LWC Component:
HTML:
<template>
<lightning-card>
Record Id is {recordId}
<br/>
Sample value is {strSample}
</lightning-card>
</template>
JavaScript:
import { LightningElement, wire, api } from 'lwc';
import { CurrentPageReference } from 'lightning/navigation';
export default class SampleAccountLWC extends LightningElement {
strSample;
@api recordId;
@wire(CurrentPageReference)
currentPageReference;
connectedCallback() {
console.log( 'Param ' + this.currentPageReference.state.c__sample );
this.strSample = this.currentPageReference.state.c__sample;
}
}
meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>50.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__RecordPage</target>
</targets>
</LightningComponentBundle>
Output:
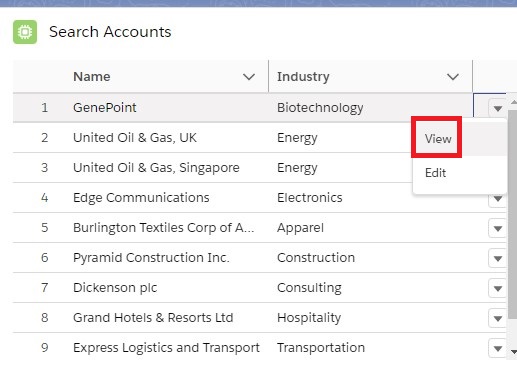
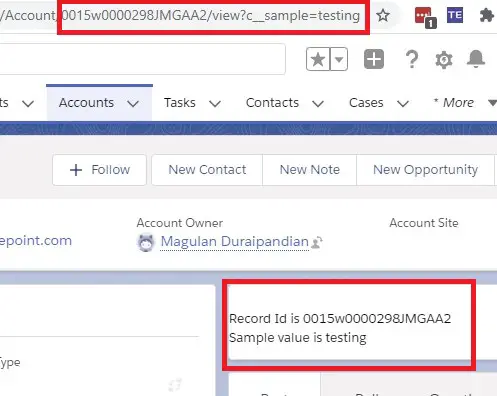