Sample Push Topic:
PushTopic pushTopic = new PushTopic();
pushTopic.Name = 'AccountUpdates';
pushTopic.Query = 'SELECT Id, Name, Phone FROM Account WHERE BillingCity=\'San Francisco\'';
pushTopic.ApiVersion = 48.0;
insert pushTopic;
Sample Lightning Web Component:
HTML:
<template>
<lightning-card title="EmpApi Example" icon-name="custom:custom14">
<div class="slds-m-around_medium">
<p>Use the buttons below to Subscribe and Unsubscribe!!!</p>
<lightning-input label="Channel Name" value={channelName}
onchange={handleChannelName}></lightning-input><br/>
<lightning-button variant="success" label="Subscribe" title="Subscribe"
onclick={handleSubscribe} disabled={isSubscribeDisabled}
class="slds-m-left_x-small"></lightning-button>
<lightning-button variant="destructive" label="Unsubscribe" title="Unsubscribe"
onclick={handleUnsubscribe} disabled={isUnsubscribeDisabled}
class="slds-m-left_x-small"></lightning-button><br/><br/>
<b>Response:</b> <br/>{strResponse}
</div>
</lightning-card>
</template>
JavaScript:
import { LightningElement, track } from 'lwc';
import { subscribe, unsubscribe, onError, setDebugFlag, isEmpEnabled } from 'lightning/empApi';
export default class PushTopicLWC extends LightningElement {
@track channelName = '/topic/AccountUpdates';
@track isSubscribeDisabled = false;
@track isUnsubscribeDisabled = !this.isSubscribeDisabled;
@track strResponse = '';
subscription = {};
// Tracks changes to channelName text field
handleChannelName(event) {
this.channelName = event.target.value;
}
// Handles subscribe button click
handleSubscribe() {
// Callback invoked whenever a new event message is received
const messageCallback = ( response ) => {
console.log( 'New message received : ', JSON.stringify( response ) );
// Response contains the payload of the new message received
this.strResponse = JSON.stringify( response );
console.log( this.strResponse );
};
// Invoke subscribe method of empApi. Pass reference to messageCallback
subscribe( this.channelName, -1, messageCallback ).then(response => {
// Response contains the subscription information on successful subscribe call
console.log( 'Successfully subscribed to : ', JSON.stringify( response.channel ) );
this.subscription = response;
this.toggleSubscribeButton( true );
});
}
// Handles unsubscribe button click
handleUnsubscribe() {
this.toggleSubscribeButton( false );
// Invoke unsubscribe method of empApi
unsubscribe(this.subscription, response => {
console.log( 'unsubscribe() response: ', JSON.stringify( response ) );
// Response is true for successful unsubscribe
});
}
toggleSubscribeButton( enableSubscribe ) {
this.isSubscribeDisabled = enableSubscribe;
this.isUnsubscribeDisabled = !enableSubscribe;
}
registerErrorListener() {
// Invoke onError empApi method
onError(error => {
console.log( 'Received error from server: ', JSON.stringify( error ) );
// Error contains the server-side error
});
}
}
JS-Meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>48.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__Tab</target>
</targets>
</LightningComponentBundle>
Output:
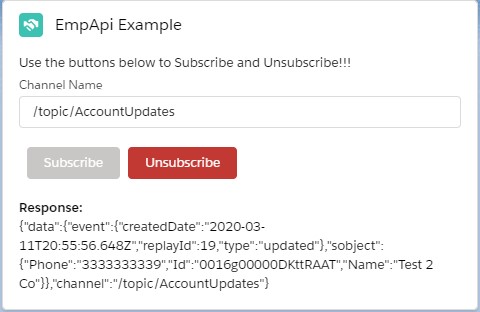