Sample Code:
Apex Class:
public with sharing class AccountController {
@AuraEnabled( cacheable = true )
public static List< Account > fetchAccounts() {
return [ SELECT Id, Name, Industry, AccountNumber, Rating, Type, Phone
FROM Account
LIMIT 100 ];
}
}
Lightning Web Component:
HTML:
<template>
<lightning-card title = "Accounts" icon-name = "custom:custom63">
<div class = "slds-m-around_medium">
<template if:true = {accounts}>
<div style="height: 300px;">
<lightning-datatable key-field="Id"
data={accounts}
columns={columns}
show-row-number-column="true"
onrowselection={handleSelect}>
</lightning-datatable>
</div>
</template>
<template if:true = {error}>
{error}>
</template>
</div>
</lightning-card>
</template>
JavaScript:
import { LightningElement, wire } from 'lwc';
import fetchAccounts from '@salesforce/apex/AccountController.fetchAccounts';
const columns = [
{ label: 'Name', fieldName: 'Name' },
{ label: 'Industry', fieldName: 'Industry' }
];
export default class sample extends LightningElement() {
accounts;
error;
columns = columns;
selectedRows = [];
@wire( fetchAccounts )
wiredAccount( value ) {
this.wiredRecords = value; // track the provisioned value
const { data, error } = value;
if ( data ) {
this.accounts = data;
this.error = undefined;
} else if ( error ) {
this.error = error;
this.accounts = undefined;
}
}
handleSelect( event ) {
const selRows = event.detail.selectedRows;
console.log( 'Selected Rows are ' + JSON.stringify ( selRows ) );
if ( this.selectedRows.length < selRows.length ) {
console.log( 'Selected' );
} else {
console.log( 'Deselected' );
let deselectedRecs = this.selectedRows
.filter(x => !selRows.includes(x))
.concat(selRows.filter(x => !this.selectedRows.includes(x)));
console.log( 'Deselected Recs are ' + JSON.stringify( deselectedRecs ) );
}
this.selectedRows = selRows;
}
}
Output:
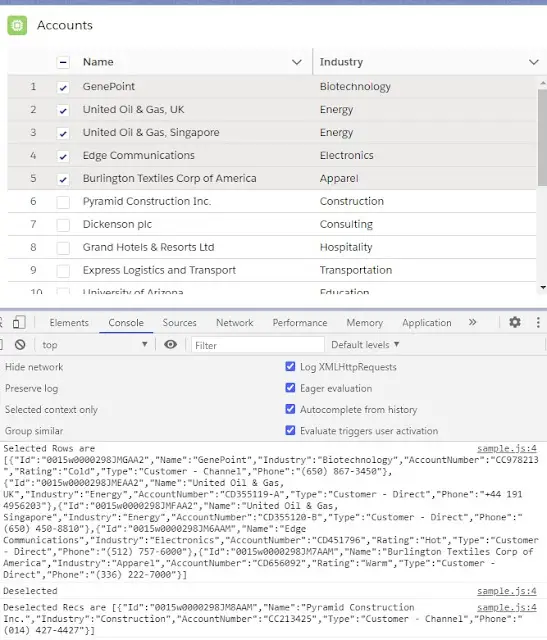