Sample Code:
HTML:
<template>
<div class="slds-p-around_medium lgc-bg">
<div class="slds-m-bottom_small">
<lightning-button label="Expand All"
onclick={clickToExpandAll}>
</lightning-button>
<lightning-button label="Collapse All"
onclick={clickToCollapseAll}>
</lightning-button>
</div> <lightning-tree-grid columns={gridColumns}
data={gridData}
key-field="Id"
hide-checkbox-column=true>
</lightning-tree-grid>
</div>
</template>
JavaScript:
import { LightningElement, track, wire } from 'lwc';
import fetchAccounts from '@salesforce/apex/ExampleController.fetchAccounts';
export default class Example extends LightningElement {
@track gridColumns = [{
type: 'text',
fieldName: 'Name',
label: 'Name'
},
{
type: 'text',
fieldName: 'Industry',
label: 'Industry'
},
{
type: 'text',
fieldName: 'FirstName',
label: 'FirstName'
},
{
type: 'text',
fieldName: 'LastName',
label: 'LastName'
},
{
type: 'text',
fieldName: 'OpptyName',
label: 'Opportunity Name'
},
{
type: 'text',
fieldName: 'StageName',
label: 'Opportunity Status'
}];
@track gridData;
@wire(fetchAccounts)
accountTreeData({ error, data }) {
console.log( 'Inside wire' );
if ( data ) {
let tempData = JSON.parse( JSON.stringify( data ) );
for ( let i = 0; i < tempData.length; i++ ) {
let cons = tempData[ i ][ "Contacts" ];
delete tempData[ i ][ "Contacts" ];
let childRecords = cons ? cons : [];
let opps = tempData[ i ][ "Opportunities" ];
for ( let opp in opps ) {
opps[ opp ].OpptyName = opps [ opp ].Name;
delete opps [ opp ].Name;
childRecords.push( opps[ opp ] );
}
delete tempData[ i ][ "Opportunities" ];
console.log( 'Child Records ' + JSON.stringify( childRecords ) );
tempData[ i ]._children = childRecords;
}
console.log( 'Final Data ' + JSON.stringify( tempData ) );
this.gridData = tempData;
} else if ( error ) {
if ( Array.isArray( error.body ) )
console.log( 'Error is ' + error.body.map( e => e.message ).join( ', ' ) );
else if ( typeof error.body.message === 'string' )
console.log( 'Error is ' + error.body.message );
}
}
clickToExpandAll( e ) {
const grid = this.template.querySelector( 'lightning-tree-grid' );
grid.expandAll();
}
clickToCollapseAll( e ) {
const grid = this.template.querySelector( 'lightning-tree-grid' );
grid.collapseAll();
}
}
Apex Class:
public with sharing class ExampleController {
@AuraEnabled(cacheable=true)
public static List < Account > fetchAccounts() {
return [ SELECT Id, Name, Industry,
( SELECT Id, FirstName, LastName FROM Contacts ),
( SELECT Id, Name, StageName FROM Opportunities ) FROM Account LIMIT 5 ];
}
}
Output:
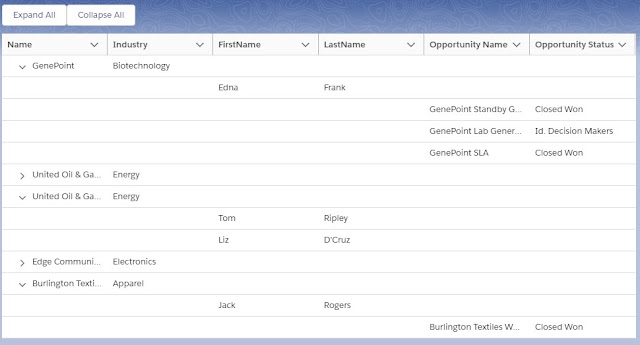