Sample Code:
Aura Component:
<aura:component implements="force:lightningQuickAction,force:hasRecordId">
<c:caseEscalation recId="{!v.recordId}"/>
</aura:component>
Lightning Web Component(LWC):
LWC HTML:
<template>
<div class="slds-m-around_medium">
<template if:true={newBool}>
<lightning-record-edit-form object-api-name="Case"
onsuccess={handleCreate}
record-type-id="0123i000000IC1dAAG">
<lightning-messages></lightning-messages>
<div class="slds-grid slds-wrap">
<div class="slds-col slds-size_1-of-2">
<lightning-input-field field-name="Subject">
</lightning-input-field>
</div>
<div class="slds-col slds-size_1-of-2">
<lightning-input-field field-name="Status">
</lightning-input-field>
</div>
<div class="slds-col slds-size_1-of-2">
<lightning-input-field field-name="Reason">
</lightning-input-field>
</div>
<div class="slds-col slds-size_1-of-2">
<lightning-input-field field-name="Origin">
</lightning-input-field>
</div>
<div class="slds-col slds-size_1-of-2">
<lightning-input-field field-name="ParentId" value={recId}>
</lightning-input-field>
</div>
</div>
<lightning-button class="slds-m-top_small"
type="submit"
label="Create new">
</lightning-button>
</lightning-record-edit-form>
</template>
<template if:true={parentCaseId}>
<template if:true={viewBool}>
<lightning-record-view-form record-id={parentCaseId}
object-api-name="Case">
<div class="slds-grid slds-wrap">
<div class="slds-col slds-size_1-of-2">
<lightning-output-field field-name="Subject">
</lightning-output-field>
</div>
<div class="slds-col slds-size_1-of-2">
<lightning-output-field field-name="Status">
</lightning-output-field>
</div>
<div class="slds-col slds-size_1-of-2">
<lightning-output-field field-name="Reason">
</lightning-output-field>
</div>
<div class="slds-col slds-size_1-of-2">
<lightning-output-field field-name="Origin">
</lightning-output-field>
</div>
</div>
</lightning-record-view-form>
</template>
<template if:true={editBool}>
<lightning-record-edit-form record-id={parentCaseId}
object-api-name="Case"
onsuccess={handleSuccess}>
<lightning-messages>
</lightning-messages>
<div class="slds-grid slds-wrap">
<div class="slds-col slds-size_1-of-2">
<lightning-input-field field-name="Subject">
</lightning-input-field>
</div>
<div class="slds-col slds-size_1-of-2">
<lightning-input-field field-name="Status">
</lightning-input-field>
</div>
<div class="slds-col slds-size_1-of-2">
<lightning-input-field field-name="Reason">
</lightning-input-field>
</div>
<div class="slds-col slds-size_1-of-2">
<lightning-input-field field-name="Origin">
</lightning-input-field>
</div>
</div>
<lightning-button
class="slds-m-top_small"
variant="brand"
type="submit"
name="update"
label="Update">
</lightning-button>
</lightning-record-edit-form>
</template>
</template >
</div>
</template>
LWC JS:
import { LightningElement, api, wire, track } from 'lwc';
import fetchCase from '@salesforce/apex/CaseEscalationController.fetchCase';
import { ShowToastEvent } from 'lightning/platformShowToastEvent';
export default class CaseEscalation extends LightningElement {
@api recId;
@track parentCaseId;
@track viewBool;
@track editBool;
@track newBool;
@wire(fetchCase, { strRecordId: '$recId' })
caseRecord({ error, data }) {
if ( data ) {
this.parentCaseId = data.Id;
}
if ( this.parentCaseId ) {
this.newBool = false;
this.editBool = true;
} else{
this.newBool = true;
this.editBool = false;
}
this.viewBool = false;
}
handleSuccess( event ) {
const toastEvent = new ShowToastEvent({
title: 'Case Updated',
message: 'Case Updated Successfully!!!',
variant: 'success'
});
this.dispatchEvent( toastEvent );
this.viewBool = true;
this.editBool = false;
this.newBool = false;
}
handleCreate( createEvent ) {
const toastEvent = new ShowToastEvent({
title: 'Case Created',
message: 'Case Created Successfully!!!',
variant: 'success'
});
this.dispatchEvent( toastEvent );
this.viewBool = true;
this.editBool = false;
this.newBool = false;
this.parentCaseId = createEvent.detail.id;
}
}
LWC JS-META.XML:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata" fqn="CaseEscalation">
<apiVersion>47.0</apiVersion>
<isExposed>false</isExposed>
<targets>
<target>lightning__RecordPage</target>
</targets>
<targetConfigs>
<targetConfig targets="lightning__RecordPage">
<property name="recId" type="String" label="Record Id" description="Record Id"/>
</targetConfig>
</targetConfigs>
</LightningComponentBundle>
Apex Class:
public class CaseEscalationController {
@AuraEnabled( cacheable=true )
public static Case fetchCase( String strRecordId ) {
system.debug( 'Id is ' + strRecordId );
List < Case > listCases = [ SELECT Id FROM Case WHERE ParentId =: strRecordId LIMIT 1 ];
if( listCases.size() > 0 )
return listCases.get( 0 );
else
return null;
}
}
Output:
Case without already created Escalation Case(Case not tied to the viewing Case through Parent Case)
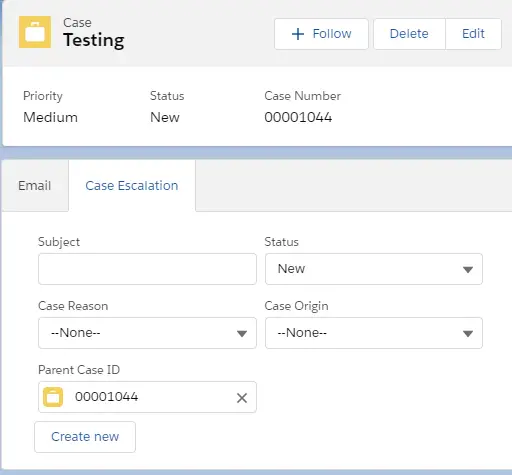
Case with already created Escalation Case(Case tied to the viewing Case through Parent Case)
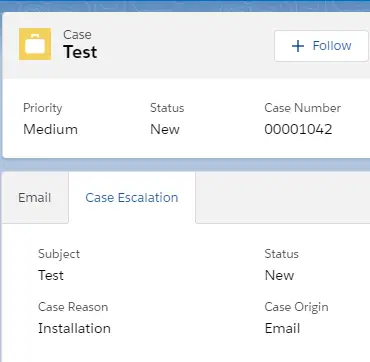